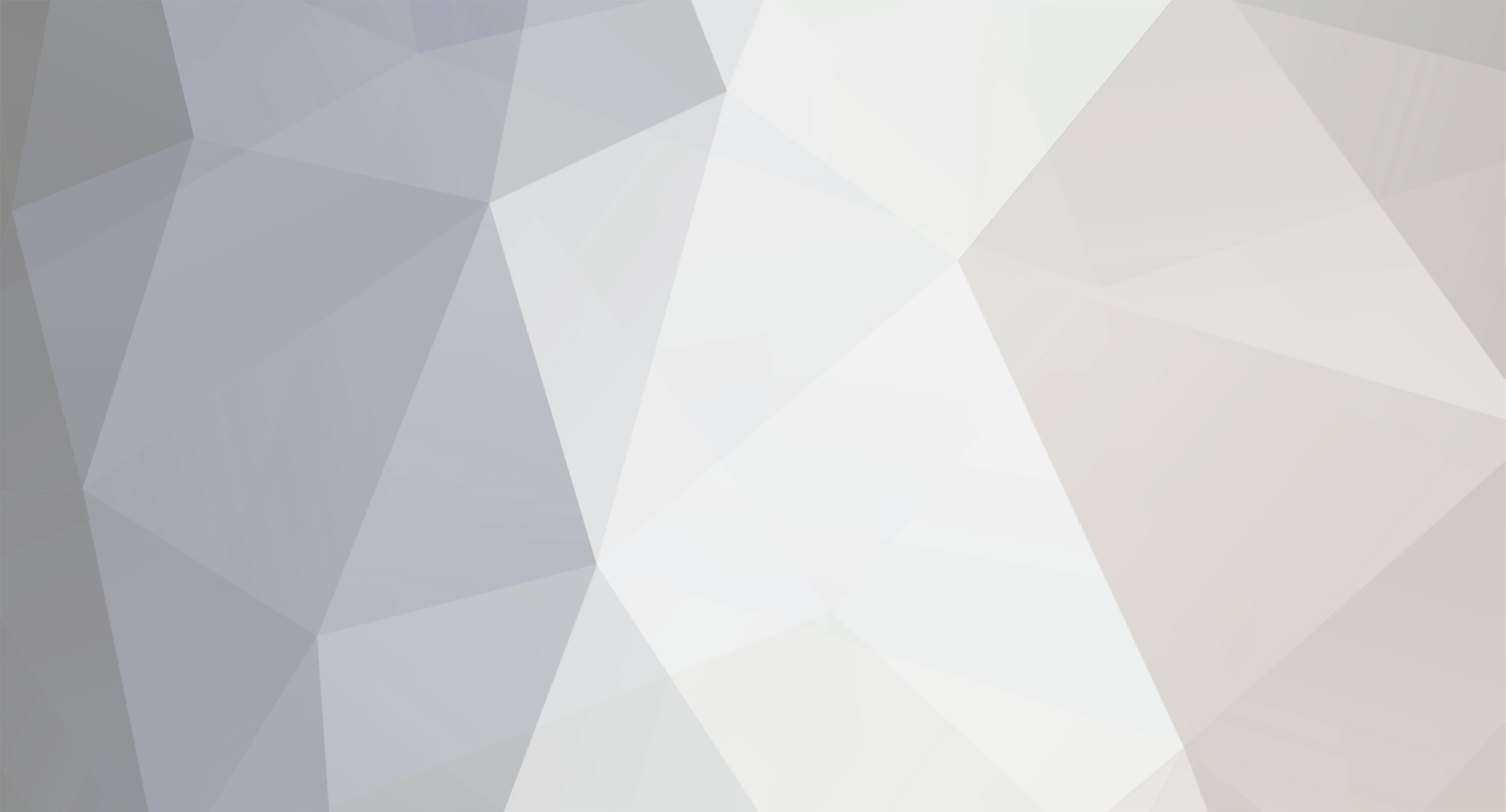
enddo
-
Posts
3 -
Joined
-
Last visited
-
Days Won
1
Posts posted by enddo
-
-
CJExploiter
CJExploiter is drag and drop ClickJacking exploit development assistance tool. First open the "index.html" with your browser locally and enter target URL and click on "View Site". You can dynamically create your own inputs. Finally by click the "Exploit It" you can see the P0C.
Feel free to make pull requests, if there's anything you feel we could do better.
Summery
Clickjacking, also known as a "UI redress attack", is when an attacker uses multiple transparent or opaque layers to trick a user into clicking on a button or link on another page when they were intending to click on the the top level page. Thus, the attacker is "hijacking" clicks meant for their page and routing them to another page, most likely owned by another application, domain, or both.
Using a similar technique, keystrokes can also be hijacked. With a carefully crafted combination of stylesheets, iframes, and text boxes, a user can be led to believe they are typing in the password to their email or bank account, but are instead typing into an invisible frame controlled by the attacker. OWASP
You can use this tool to generate dynamic P0C.
-
3
-
-
A curated list of awesome Windows Exploitation resources, and shiny things.
Table of Contents
- Windows stack overflows
- Windows heap overflows
- Kernel based Windows overflows
- Windows memory protections
- Bypassing filter and protections
- Typical windows exploits
- Exploit development tutorial series
- Tools
Windows stack overflows
Stack Base Overflow Articles.
- Win32 Buffer Overflows (Location, Exploitation and Prevention) - by dark spyrit [1999]
- Writing Stack Based Overflows on Windows - by Nish Bhalla’s [2005]
Windows heap overflows
Heap Base Overflow Articles.
- Third Generation Exploitation smashing heap on 2k - by halvar Flake [2002]
- Exploiting the MSRPC Heap Overflow Part 1 - by Dave Aitel (MS03-026) [September 2003]
- Exploiting the MSRPC Heap Overflow Part 2 - by Dave Aitel (MS03-026) [September 2003]
- windows heap overflow penetration in black hat - by David litchfield [2004]
Kernel based Windows overflows
Kernel Base Exploit Development Articles.
- how to attack kernel based vulns on windows was done - by a Polish group called “sec-labs” [2003]
- sec-lab old whitepaper
- sec-lab old exploit
- Windows Local Kernel Exploitation (based on sec-lab research) - by S.K Chong [2004]
- How to exploit Windows kernel memory pool - by SoBeIt [2005]
- exploiting remote kernel overflows in windows - by eeye security
- Kernel-mode Payloads on Windows in uninformed - by matt miller
- Exploiting 802.11 Wireless Driver Vulnerabilities on Windows
- BH US 2007 Attacking the Windows Kernel
- Remote and Local Exploitation of Network Drivers
- Exploiting Comon Flaws In Drivers
- I2OMGMT Driver Impersonation Attack
- Real World Kernel Pool Exploitation
- exploit for windows 2k3 and 2k8
- nalyzing local privilege escalations in win32k
- Intro to Windows Kernel Security Development
- There’s a party at ring0 and you’re invited
- Windows kernel vulnerability exploitation
Windows memory protections
Windows memory protections Introduction Articles.
Bypassing filter and protections
Windows memory protections Bypass Methods Articles.
- Third Generation Exploitation smashing heap on 2k - by halvar Flake [2002]
- chris anley wrote Creating Arbitrary Shellcode In Unicode Expanded Strings
- Dave aitel advanced windows exploitation - [2003]
- Defeating the Stack Based Buffer Overflow Prevention Mechanism of Microsoft Windows 2003 Server - by david litchfield
- reliable heap exploits and after that Windows Heap Exploitation (Win2KSP0 through WinXPSP2) - by matt Conover in cansecwest 2004
- Safely Searching Process Virtual Address Space - by matt miller [2004]
- IE exploit and used a technology called Heap Spray
- bypassing hardware-enforced DEP - by skape (matt miller) and Skywing (ken johnson) [October 2005]
- Exploiting Freelist[0] On XP Service Pack 2 - by brett moore [2005]
- Kernel-mode Payloads on Windows in uninformed
- Exploiting 802.11 Wireless Driver Vulnerabilities on Windows
- Exploiting Comon Flaws In Drivers
- Heap Feng Shui in JavaScript by Alexander sotirov [2007]
- Understanding and bypassing Windows Heap Protection - by Nicolas Waisman [2007]
- Heaps About Heaps - by brett moore [2008]
- Bypassing browser memory protections in Windows Vista - by Mark Dowd and Alex Sotirov [2008]
- Attacking the Vista Heap - by ben hawkes [2008]
- Return oriented programming Exploitation without Code Injection - by Hovav Shacham (and others ) [2008]
- Token Kidnapping and a super reliable exploit for windows 2k3 and 2k8 - by Cesar Cerrudo [2008]
- Defeating DEP Immunity Way - by Pablo sole [2008]
- Practical Windows XP2003 Heap Exploitation - by John McDonald and Chris Valasek [2009]
- Bypassing SEHOP - by Stefan Le Berre Damien Cauquil [2009]
- Interpreter Exploitation : Pointer Inference and JIT Spraying - by Dionysus Blazakis[2010]
- write-up of Pwn2Own 2010 - by Peter Vreugdenhil
- all in one 0day presented in rootedCON - by ruben santamarta [2010]
- DEP/ASLR bypass using 3rd party - by Shahin Ramezany [2013]
Typical windows exploits
- real-world HW-DEP bypass Exploit - by devcode
- bypassing DEP by returning into HeapCreate - by toto
- first public ASLR bypass exploit by using partial overwrite - by skape
- heap spray and bypassing DEP - by skylined
- first public exploit that used ROP for bypassing DEP in adobe lib TIFF vulnerability
- exploit codes of bypassing browsers memory protections
- PoC’s on Tokken TokenKidnapping . PoC for 2k3 -part 1 - by Cesar Cerrudo
- PoC’s on Tokken TokenKidnapping . PoC for 2k8 -part 2 - by Cesar Cerrudo
- an exploit works from win 3.1 to win 7 - by Tavis Ormandy KiTra0d
- old ms08-067 metasploit module multi-target and DEP bypass
- PHP 6.0 Dev str_transliterate() Buffer overflow – NX + ASLR Bypass
- SMBv2 Exploit - by Stephen Fewer
Exploit development tutorial series
Exploid Development Tutorial Series Base on Windows Operation System Articles.
-
Corelan Team
- Exploit writing tutorial part 1 : Stack Based Overflows
- Exploit writing tutorial part 2 : Stack Based Overflows – jumping to shellcode
- Exploit writing tutorial part 3 : SEH Based Exploits
- Exploit writing tutorial part 3b : SEH Based Exploits – just another example
- Exploit writing tutorial part 4 : From Exploit to Metasploit – The basics
- Exploit writing tutorial part 5 : How debugger modules & plugins can speed up basic exploit development
- Exploit writing tutorial part 6 : Bypassing Stack Cookies, SafeSeh, SEHOP, HW DEP and ASLR
- Exploit writing tutorial part 7 : Unicode – from 0x00410041 to calc
- Exploit writing tutorial part 8 : Win32 Egg Hunting
- Exploit writing tutorial part 9 : Introduction to Win32 shellcoding
- Exploit writing tutorial part 10 : Chaining DEP with ROP – the Rubik’s Cube
- Exploit writing tutorial part 11 : Heap Spraying Demystified
-
Fuzzysecurity
- Part 1: Introduction to Exploit Development
- Part 2: Saved Return Pointer Overflows
- Part 3: Structured Exception Handler (SEH)
- Part 4: Egg Hunters
- Part 5: Unicode 0x00410041
- Part 6: Writing W32 shellcode
- Part 7: Return Oriented Programming
- Part 8: Spraying the Heap [Chapter 1: Vanilla EIP]
- Part 9: Spraying the Heap [Chapter 2: Use-After-Free]
-
Securitysift
- Windows Exploit Development – Part 1: The Basics
- Windows Exploit Development – Part 2: Intro to Stack Based Overflows
- Windows Exploit Development – Part 3: Changing Offsets and Rebased Modules
- Windows Exploit Development – Part 4: Locating Shellcode With Jumps
- Windows Exploit Development – Part 5: Locating Shellcode With Egghunting
- Windows Exploit Development – Part 6: SEH Exploits
- Windows Exploit Development – Part 7: Unicode Buffer Overflows
Tools
Disassemblers, debuggers, and other static and dynamic analysis tools.
- angr - Platform-agnostic binary analysis framework developed at UCSB's Seclab.
- BARF - Multiplatform, open source Binary Analysis and Reverse engineering Framework.
- binnavi - Binary analysis IDE for reverse engineering based on graph visualization.
- Bokken - GUI for Pyew and Radare.
- Capstone - Disassembly framework for binary analysis and reversing, with support for many architectures and bindings in several languages.
- codebro - Web based code browser using clang to provide basic code analysis.
- dnSpy - .NET assembly editor, decompiler and debugger.
- Evan's Debugger (EDB) - A modular debugger with a Qt GUI.
- GDB - The GNU debugger.
- GEF - GDB Enhanced Features, for exploiters and reverse engineers.
- hackers-grep - A utility to search for strings in PE executables including imports, exports, and debug symbols.
- IDA Pro - Windows disassembler and debugger, with a free evaluation version.
- Immunity Debugger - Debugger for malware analysis and more, with a Python API.
- ltrace - Dynamic analysis for Linux executables.
- objdump - Part of GNU binutils, for static analysis of Linux binaries.
- OllyDbg - An assembly-level debugger for Windows executables.
- PANDA - Platform for Architecture-Neutral Dynamic Analysis
- PEDA - Python Exploit Development Assistance for GDB, an enhanced display with added commands.
- pestudio - Perform static analysis of Windows executables.
- Process Monitor - Advanced monitoring tool for Windows programs.
- Pyew - Python tool for malware analysis.
- Radare2 - Reverse engineering framework, with debugger support.
- SMRT - Sublime Malware Research Tool, a plugin for Sublime 3 to aid with malware analyis.
- strace - Dynamic analysis for Linux executables.
- Udis86 - Disassembler library and tool for x86 and x86_64.
- Vivisect - Python tool for malware analysis.
- X64dbg - An open-source x64/x32 debugger for windows.
-
1
HatDBG
in Programe hacking
Posted
The HatDBG is A pure Powershell win32 debugging abstraction class.The goal of this project is to make a powershell debugger. This is exclusively for educational purposes.
URL: https://github.com/enddo/HatDBG
Enumerate Threads
Output
Get Debug Event Code
Output
Set Breakpoint
Output